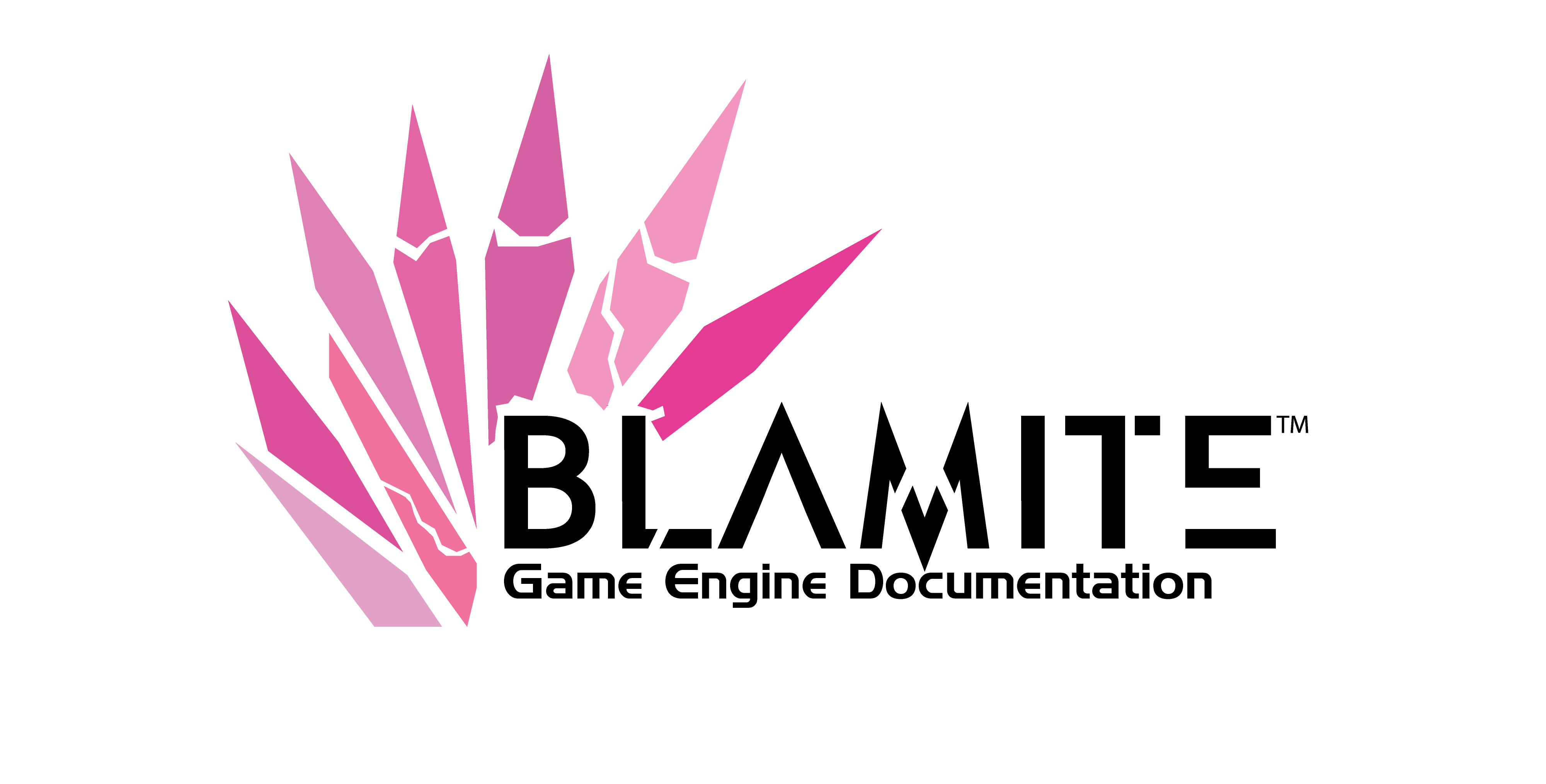 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
8 #include <Strings/components/utils/saferelease/saferelease.h>
49 namespace MFFontGlobals
126 virtual HRESULT __stdcall
QueryInterface(REFIID iid,
void** ppvObject);
131 virtual ULONG __stdcall
AddRef();
136 virtual ULONG __stdcall
Release();
152 virtual HRESULT __stdcall
CreateEnumeratorFromKey(IDWriteFactory* factory,
void const* collectionKey, UINT32 collectionKeySize, IDWriteFontFileEnumerator** fontFileEnumerator);
155 ULONG reference_count;
179 HRESULT
Initialize(UINT
const* collectionKey, UINT32 keySize);
186 SafeRelease(¤t_file);
198 virtual HRESULT __stdcall
QueryInterface(REFIID iid,
void** ppvObject);
203 virtual ULONG __stdcall
AddRef();
208 virtual ULONG __stdcall
Release();
221 virtual HRESULT __stdcall
MoveNext(BOOL* hasCurrentFile);
233 ULONG reference_count;
235 IDWriteFontFile* current_file;
236 std::vector<std::wstring> file_paths;
MFFontCollectionLoader()
Sets the reference count to 0.
Definition: FontLoader.h:112
#define BLAM
Definition: FontLoader.h:11
HRESULT Initialize(UINT const *collectionKey, UINT32 keySize)
Initializes a the enumerator.
Definition: MFFontFileEnumerator.cpp:16
virtual HRESULT __stdcall GetCurrentFontFile(IDWriteFontFile **fontFile)
Retrieves the current font file.
Definition: MFFontFileEnumerator.cpp:91
Namespace containing things relating to the MF font loader.
Definition: FontLoader.h:44
virtual ULONG __stdcall Release()
Releases the object.
Definition: MFFontFileEnumerator.cpp:56
virtual HRESULT __stdcall QueryInterface(REFIID iid, void **ppvObject)
Queries the interface.
Definition: MFFontFileEnumerator.cpp:36
virtual HRESULT __stdcall QueryInterface(REFIID iid, void **ppvObject)
Queries the interface.
Definition: MFFontCollectionLoader.cpp:5
~MFFontFileEnumerator()
Releases the current file in the enumerator.
Definition: FontLoader.h:184
virtual HRESULT __stdcall CreateEnumeratorFromKey(IDWriteFactory *factory, void const *collectionKey, UINT32 collectionKeySize, IDWriteFontFileEnumerator **fontFileEnumerator)
Creates a new Font File Enumerator.
Definition: MFFontCollectionLoader.cpp:37
MFFontFileEnumerator(IDWriteFactory *factory)
Sets reference counts and indexes to 0.
Definition: MFFontFileEnumerator.cpp:9
virtual HRESULT __stdcall MoveNext(BOOL *hasCurrentFile)
Moves to the next item in the enumerator.
Definition: MFFontFileEnumerator.cpp:68
virtual ULONG __stdcall AddRef()
Acquires a new reference to the object.
Definition: MFFontFileEnumerator.cpp:51
BLAM std::vector< MFFontList > & getFontFileLists()
Retrieves the list of font lists.
Definition: FontLoader.cpp:95
std::vector< std::wstring > MFFontList
Somewhat pointless font list type definition, but it does make things slightly easier to read.
Definition: FontLoader.h:17
virtual ULONG __stdcall AddRef()
Acquires a new reference to the object.
Definition: MFFontCollectionLoader.cpp:20
BLAM void addFontCollection(std::string id, IDWriteFontCollection *collection)
Adds a font collection to the list of font collections.
Definition: FontLoader.cpp:65
virtual ULONG __stdcall Release()
Releases the object.
Definition: MFFontCollectionLoader.cpp:25
BLAM HRESULT CreateFontCollection(MFFontList &newCollection, std::string font_id)
Creates a new font collection.
Definition: FontLoader.cpp:26
Class to implement the DirectWrite Font File Enumerator interface.
Definition: FontLoader.h:163
BLAM int push(MFFontList &collection)
Inserts a font list into the list of font lists.
Definition: FontLoader.cpp:56
Class to assist in the loading of font collections.
Definition: FontLoader.h:106
BLAM IDWriteFontCollection * getFontCollection(std::string id)
Retrieves a font collection from the list of font collections.
Definition: FontLoader.cpp:70
BLAM void cleanupCollectionList()
Cleans up the font collection list.
Definition: FontLoader.cpp:85