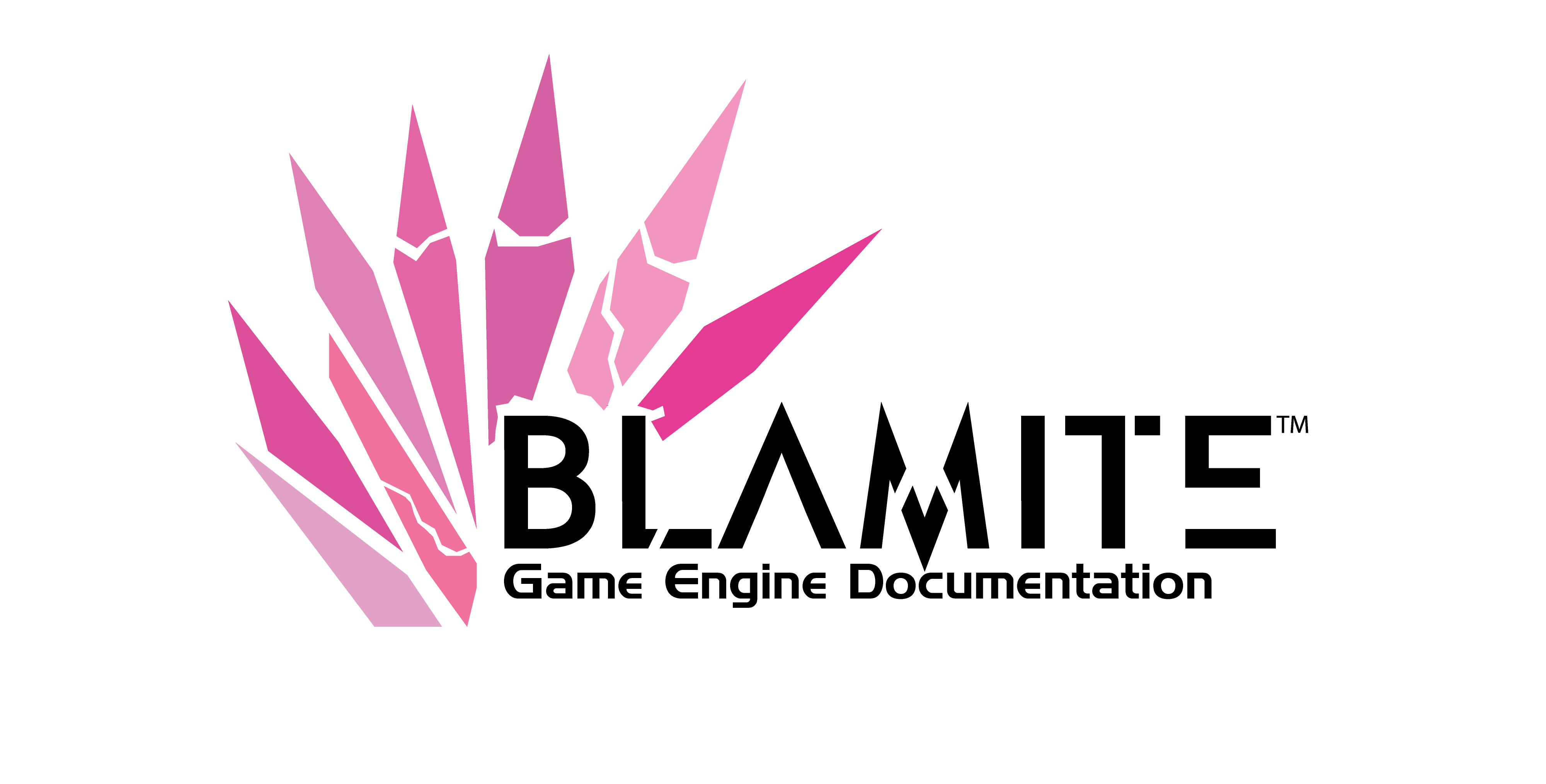 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
12 #define FONTINFO_FILENAME "fontinfo.xml"
13 #define FONT_PACKAGE_EXTENSION ".bin"
14 #define FONT_PACKAGE_VERSION 2
Namespace surrounding all major engine components.
Definition: blam_api.h:18
BLAM HRESULT ReloadFont(std::string id)
Reloads all data for the specified font.
Definition: fonts.cpp:867
FontGlyphFormat format
The format of the glyph image data. See FontGlyphFormat for more details.
Definition: fonts.h:57
BLAM bool FontExists(std::string id)
Checks whether or not a font is loaded.
Definition: fonts.cpp:26
std::string ttf_path
The path to the TrueType font file.
Definition: fonts.h:85
int ttf_offset
The offset of the embedded TrueType font data within the font package.
Definition: fonts.h:73
std::string package_engine_version
The minimum engine version to support the font package version specified.
Definition: fonts.h:71
#define BLAM
Definition: fonts.h:9
BLAM std::map< std::string, Font > * GetFontList()
Retrieves the list of loaded fonts.
Definition: fonts.cpp:835
BLAM void Cleanup()
Clean-up all font data and free any allocated memory for font data.
Definition: fonts.cpp:887
BLAM Font * GetFont(std::string id)
Retrieves a font from the list of loaded fonts.
Definition: fonts.cpp:806
short charspacing
The amount of space, in pixels, between each character.
Definition: fonts.h:78
int data_length
The length of the glyph's image data. Only used in the case of a font package.
Definition: fonts.h:49
BLAM HRESULT LoadFont(std::string path)
Load a font from disk.
Definition: fonts.cpp:46
std::string file_path
The path of the glyph's image file. Only used in the case of an unpackaged font.
Definition: fonts.h:59
int data_offset
The file offset of the glyph's raw image data. Only used in the case of a font package.
Definition: fonts.h:48
bool is_truetype
Whether or not the font is a TrueType based font.
Definition: fonts.h:84
Structure to contain data for a Font Glyph.
Definition: fonts.h:45
FontGlyphFormat
Enumerator for the image format used in a given font glyph.
Definition: fonts.h:24
int ttf_length
The length of the embedded TrueType font data.
Definition: fonts.h:74
std::map< char, FontGlyph > glyph_list
The list of glyphs for this font.
Definition: fonts.h:97
@ PNG
Indicates the glyph originates from a .PNG image.
Definition: fonts.h:26
short size
The size of the font.
Definition: fonts.h:77
bool monospaced
Whether or not to treat the font as a monospace font.
Definition: fonts.h:79
short mono_width
The width for each character to be. Extra space will be left as needed if the glyph width is too smal...
Definition: fonts.h:80
Structure to contain data for a Font.
Definition: fonts.h:68
bool is_font_package
Whether or not the data originates from a font package.
Definition: fonts.h:72
std::string path
The path of the font package, excluding the file extension.
Definition: fonts.h:88
std::string file_path
The path to either the font package or the fontinfo.xml file for this font.
Definition: fonts.h:89
short width
The width of the glyph image, in pixels.
Definition: fonts.h:55
void * bitmap_data
The raw image data loaded from a font package. This must be available for the lifetime of the font,...
Definition: fonts.h:62
short space_width
The amount of space, in pixels, to use for the space character.
Definition: fonts.h:81
BLAM FontGlyph * GetFontGlyph(Font *font, char glyph)
Retrieves a glyph from a font.
Definition: fonts.cpp:820
char character
The character that the glyph represents.
Definition: fonts.h:52
std::string id
The unique ID of the font.
Definition: fonts.h:76
short line_height
The amount of space, in pixels, between each line.
Definition: fonts.h:82
std::string ttf_name
The display name of the TrueType font file. TODO: Make this shit automated.
Definition: fonts.h:86
short height
The height of the glyph image, in pixels.
Definition: fonts.h:56
IWICBitmap * bitmap
The WIC bitmap for this glyph.
Definition: fonts.h:61
std::string path
The path of the glyph's image file, relative to fontinfo.xml's location. Only used in the case of an ...
Definition: fonts.h:58
BLAM void LoadAllFonts()
Loads all fonts available in the engine's configured font directory.
Definition: fonts.cpp:900
short package_version
The version of the font package.
Definition: fonts.h:70