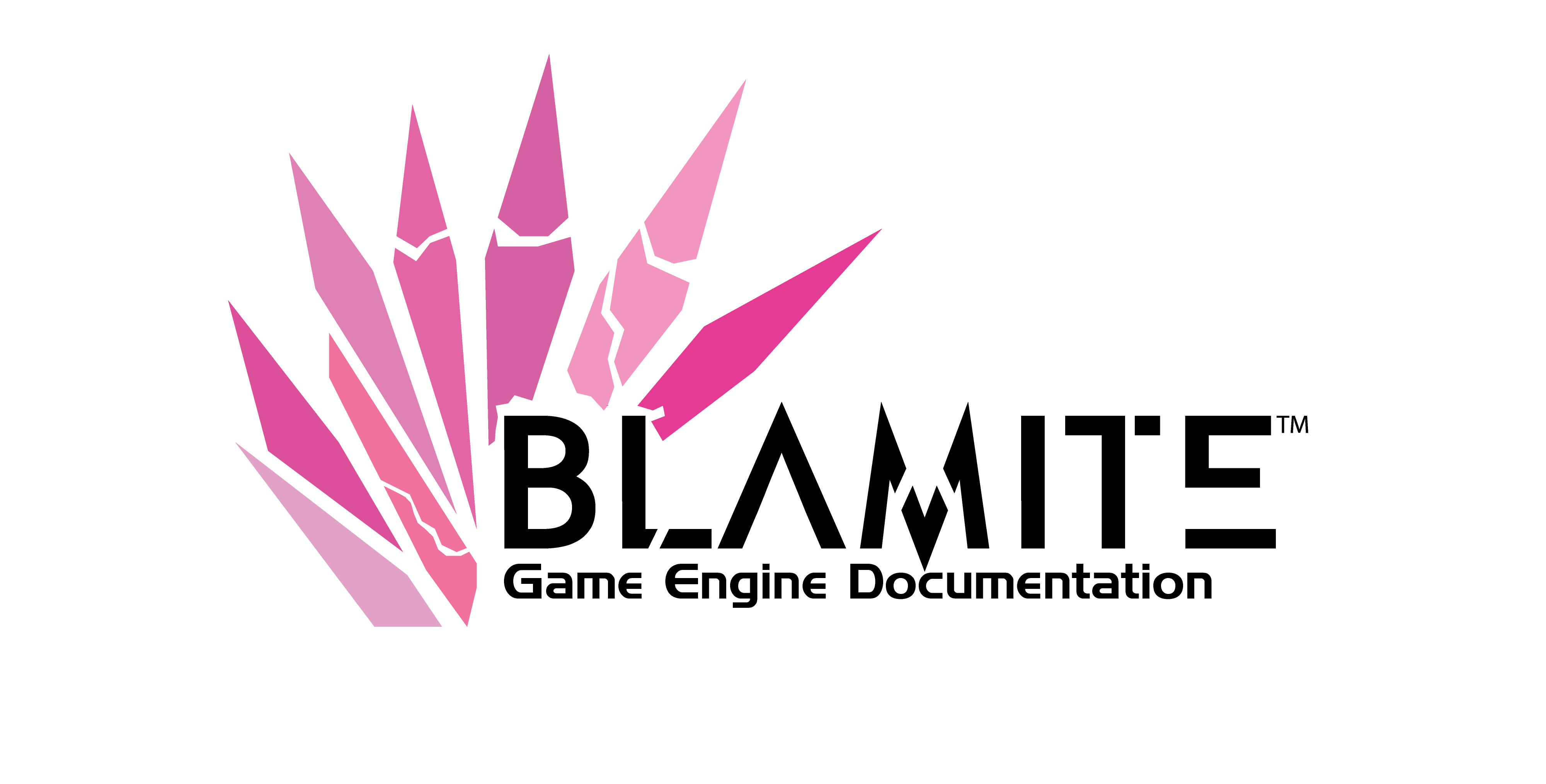 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
6 #include "../../render_stack.h"
30 fps_counter->
color = D2D1::ColorF(1, 1, 1, 1);
60 std::stringstream fps_stream;
61 fps_stream << std::fixed << std::setprecision(2) << ImGui::GetIO().Framerate;
62 std::string fps = fps_stream.str();
64 if (ImGui::GetIO().Framerate >= 30)
66 fps_counter->
color = D2D1::ColorF(0, 1, 0, 1);
68 else if (ImGui::GetIO().Framerate < 15)
70 fps_counter->
color = D2D1::ColorF(1, 0, 0, 1);
72 else if (ImGui::GetIO().Framerate < 30)
74 fps_counter->
color = D2D1::ColorF(1, 1, 0, 1);
77 fps_counter->
text =
"fps: " + fps +
"";
bool visible
Whether or not the object is visible.
Definition: render_stack.h:375
void ShowImPropertyEditor()
Shows a set of ImGUI properties associated with the object.
Definition: fpscounter.hpp:83
std::string font_id
The ID of the font.
Definition: render_stack.h:844
@ BitmapText
Bitmap-based text, uses Blamite font system.
Definition: render_stack.h:72
StackType type_label
The type of the object.
Definition: render_stack.h:372
BLAM ConfigFile * GetConfig()
Definition: compat.cpp:5
Namespace containing things relating to the Render Stack.
Definition: drawing.h:14
std::string text
The text to display.
Definition: render_stack.h:846
D2D1_COLOR_F color
The color of the object.
Definition: render_stack.h:370
void Draw()
Draws the stack object.
Definition: BitmapText.cpp:13
#define STACKTYPE_FPS_COUNTER
Definition: render_stack.h:25
bool use_shadow
Whether or not to draw the text with a drop shadow.
Definition: render_stack.h:852
void SetTranslation(float new_x, float new_y)
Sets the translation of the object.
Definition: StackObjectBase.cpp:55
void Draw()
Draws the FPS counter onscreen.
Definition: fpscounter.hpp:52
FPSCounter()
Prepares the FPS counter.
Definition: fpscounter.hpp:22
Class used for the FPS counter.
Definition: fpscounter.hpp:13
std::string GetString(std::string option)
Definition: compat.cpp:58
Class representing text drawn using a Bitmap-based engine font.
Definition: render_stack.h:794
Base class for all render stack objects.
Definition: render_stack.h:194
~FPSCounter()
Cleans up any resources used by the FPS counter.
Definition: fpscounter.hpp:40