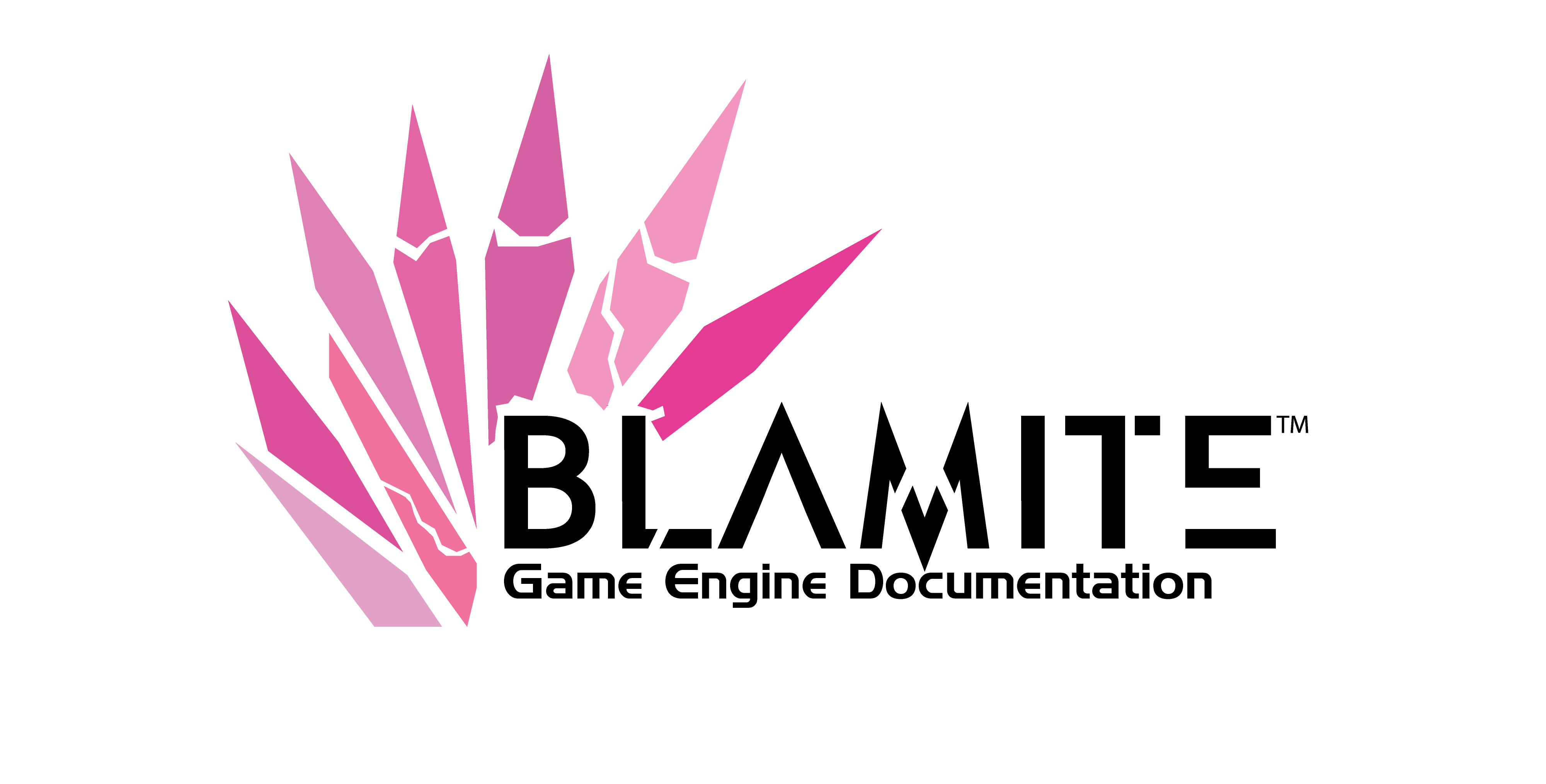 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
7 #include <Strings/components/resources/color/BlamColor.h>
8 #include <Strings/components/utils/string/string.h>
10 #define GVARS_FILE "./globals.xml"
Namespace surrounding all major engine components.
Definition: blam_api.h:18
BLAM void RegisterGvar(EngineGlobal var)
Registers a new engine global.
Definition: globals.cpp:65
BLAM EngineGlobal * GetGlobal(std::string name)
Retrieves a global with the specified ID.
Definition: globals.cpp:193
BLAM bool GlobalExists(std::string id)
Determines whether or not a global exists.
Definition: globals.cpp:27
float float_value
The float value of the global.
Definition: globals.h:78
std::string info
An optional description of the global.
Definition: globals.h:66
@ Int
Represents an int.
Definition: globals.h:47
BlamColor color_value
The color value of the global.
Definition: globals.h:79
@ UnknownGlobal
The specified global does not exist.
Definition: globals.h:29
@ Long
Represents a long.
Definition: globals.h:44
@ GlobalIsProtected
The specified global is protected and cannot be modified during runtime.
Definition: globals.h:33
BLAM bool LoadGlobalsFromFile()
Loads any globals from GVARS_FILE.
Definition: globals.cpp:206
BLAM float * GetGlobalAsFloat(std::string name)
Retrieves a global's value as a float.
Definition: globals.cpp:400
@ InvalidArgs
The provided arguments were invalid.
Definition: globals.h:31
@ Real
Same as Float.
Definition: globals.h:42
BLAM GvarUpdateResult UpdateGlobalWrap(std::string name, std::string new_value)
Updates a global's raw value.
Definition: globals.cpp:428
bool read_only
Whether or not the global is protected from modification.
Definition: globals.h:68
std::string name
The name of the global.
Definition: globals.h:65
GvarType
Enumerator for the type of global variable.
Definition: globals.h:39
short short_value
The short value of the global.
Definition: globals.h:75
BLAM BlamColor * GetGlobalAsColor(std::string name)
Retrieves a global's value as a BlamColor.
Definition: globals.cpp:412
@ String
Represents a std::string.
Definition: globals.h:46
#define BLAM
Definition: globals.h:13
Structure containing data for a game engine global.
Definition: globals.h:62
@ Float
Represents a float.
Definition: globals.h:48
long long_value
The long value of the global.
Definition: globals.h:76
GvarUpdateResult
Enumerator for the result of a global update attempt.
Definition: globals.h:26
BLAM short * GetGlobalAsShort(std::string name)
Retrieves a global's value as a short.
Definition: globals.cpp:364
@ InvalidType
The provided value was of an invalid type.
Definition: globals.h:28
@ Object
Unknown. Referenced within the hs_doc from Halo 2 Sapien.
Definition: globals.h:45
@ Short
Represents a short.
Definition: globals.h:43
int int_value
The int value of the global.
Definition: globals.h:77
BLAM std::map< std::string, EngineGlobal > * GetGlobalsList()
Retrieves the list of loaded globals.
Definition: globals.cpp:22
@ OutOfBounds
The provided value was too small or too large for the globals' data type.
Definition: globals.h:32
BLAM long * GetGlobalAsLong(std::string name)
Retrieves a global's value as a long.
Definition: globals.cpp:376
@ Color
Represents a BlamColor. See #BlamColor for details.
Definition: globals.h:49
@ Boolean
Represents a boolean. Can be true or false.
Definition: globals.h:41
BLAM std::string * GetGlobalAsString(std::string name)
Retrieves a global's value as a string.
Definition: globals.cpp:352
std::string value_raw
The raw value of the global as a string.
Definition: globals.h:67
GvarType type
The type of the global.
Definition: globals.h:64
@ Ok
The global was updated successfully.
Definition: globals.h:30
BLAM bool * GetGlobalAsBoolean(std::string name)
Retrieves a global's value as a boolean.
Definition: globals.cpp:340
bool boolean_value
The boolean value of the global.
Definition: globals.h:74
BLAM GvarUpdateResult UpdateGlobal(std::string name, std::string new_value)
Updates the value of a String global.
Definition: globals.cpp:571
BLAM std::string GetGvarTypeLabel(GvarType type)
Retrieves a string representation of a global's type, for use in UI.
Definition: globals.cpp:40
BLAM int * GetGlobalAsInteger(std::string name)
Retrieves a global's value as an int.
Definition: globals.cpp:388