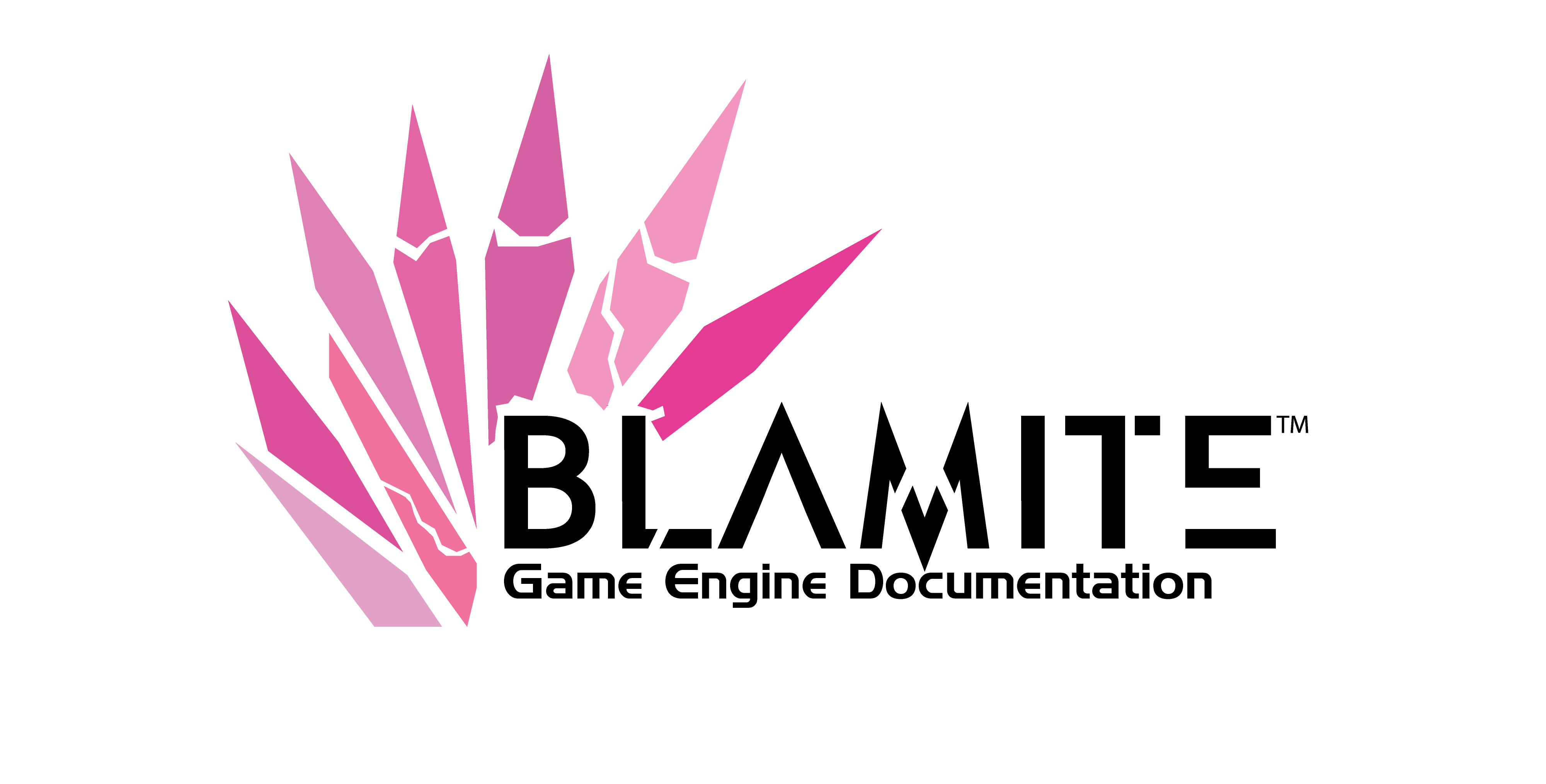 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file. 1 #include "../console.h"
3 #include <Strings/components/utils/io/io.h>
20 description =
"saves a file called hs_doc.txt with parameters for all script commands and globals.";
22 syntax =
"script_doc [quiet]";
36 if (arguments.size() > 0)
38 if (arguments[0] ==
"quiet")
44 std::string doc_contents =
"";
48 doc_contents +=
"== Built-in Commands ==";
49 doc_contents +=
"\n\n\n\n";
52 std::map<std::string, BlamConsoleCommand*>::iterator it;
54 for (it = commands.begin(); it != commands.end(); it++)
60 std::string syntax_line =
"(";
62 syntax_line += command->
name;
67 syntax_line += command->
syntax;
72 doc_contents += syntax_line;
76 if (command->
aliases.size() > 0)
78 doc_contents +=
"\n\n";
80 std::string aliases_line =
"aliases: [";
82 for (
int i = 0; i < command->
aliases.size(); i++)
84 std::string alias = command->
aliases.at(i);
86 aliases_line += alias;
88 if (i != command->
aliases.size() - 1)
96 doc_contents += aliases_line;
102 doc_contents +=
"\n\n";
108 doc_contents +=
"\n\n";
109 doc_contents +=
"NETWORK SAFE: Unspecified (networking is not available yet)";
112 doc_contents +=
"\n\n\n\n";
118 doc_contents +=
"== Commands ==";
119 doc_contents +=
"\n\n\n\n";
128 doc_contents +=
"== Script Globals ==";
129 doc_contents +=
"\n\n\n\n";
132 std::map<std::string, EngineGlobal>::iterator it;
138 std::string global_line =
"(" + it->second.name +
" <" +
GetGvarTypeLabel(it->second.type) +
">)";
140 if (it->second.read_only)
142 global_line +=
" [protected]";
145 doc_contents += global_line;
148 if (it->second.info !=
"")
150 doc_contents +=
"\n";
151 doc_contents += it->second.info;
154 doc_contents +=
"\n\n";
160 BlamStrings::Utils::IO::CreateNewFile(file_path, doc_contents);
BLAM bool OpenLocalURL(std::string url)
Definition: keystone.cpp:94
~ScriptDocCommand()
Definition: script_doc.hpp:27
ScriptDocCommand()
Definition: script_doc.hpp:17
std::string description
An optional description of the command. Shown when using the classify command.
Definition: console.h:57
BlamCommandResult onCommand(std::vector< std::string > arguments)
Called upon command execution.
Definition: script_doc.hpp:32
Namespace containing things relating to game engine globals.
Definition: globals.h:21
Class used to represent a console command.
Definition: console.h:54
BLAM ConfigFile * GetConfig()
Definition: compat.cpp:5
std::map< std::string, EngineGlobal > globals
The list of loaded globals.
Definition: globals.cpp:20
std::string name
The name of the console command.
Definition: console.h:56
BLAM std::map< std::string, BlamConsoleCommand * > GetCommandList()
Retrieves the list of all loaded console commands.
Definition: console.cpp:170
Class for the script_doc command.
Definition: script_doc.hpp:14
BLAM std::map< std::string, EngineGlobal > * GetGlobalsList()
Retrieves the list of loaded globals.
Definition: globals.cpp:22
std::string syntax
The syntax information for the command. Shown to the user when using the help command with an argumen...
Definition: console.h:58
BlamCommandType type
The type of command this is. See #Blam::Resources::Console::BlamCommandType for more information.
Definition: console.h:61
@ Builtin
A command that is hard-coded into the engine.
#define CMD_OK
Macro for OK command status. See #Blam::Resources::Console::Ok for more details.
Definition: console.h:8
BlamCommandResult
Indicates the return state of a console command.
Definition: console.h:22
std::string GetString(std::string option)
Definition: compat.cpp:58
std::vector< std::string > aliases
A list of aliases for the command. Executing any of these instead of the command name will behave the...
Definition: console.h:59
Namespace for things relating to the debug console.
Definition: abort.hpp:5
BLAM std::string GetGvarTypeLabel(GvarType type)
Retrieves a string representation of a global's type, for use in UI.
Definition: globals.cpp:40