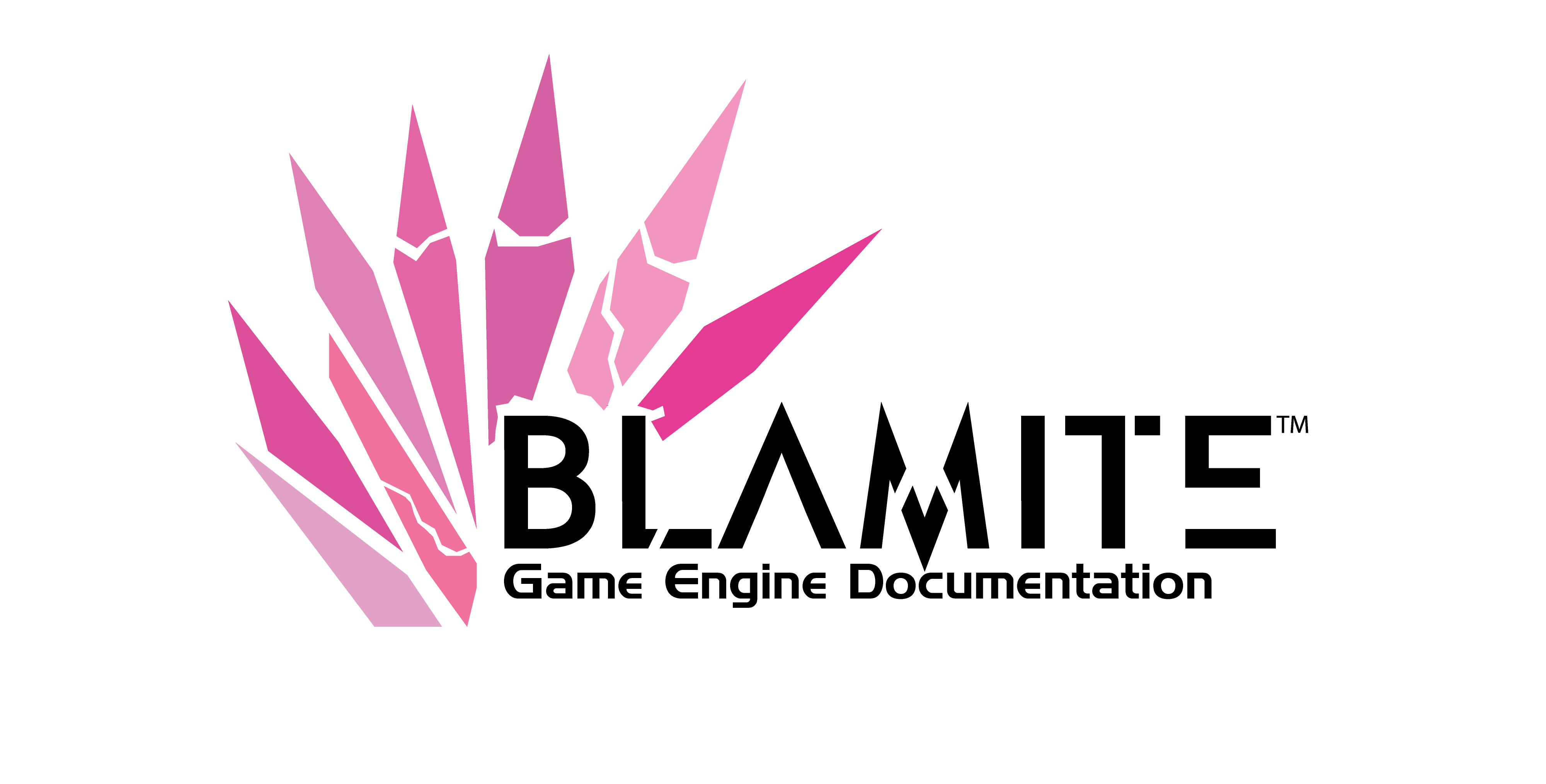 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../../debug_ui.h"
19 if (ImGui::Begin(
"Director Debug", &
show))
27 ImGui::DragFloat3(
"camera pos", (
float*)&director->
camera_pos);
28 ImGui::DragFloat3(
"camera angle", (
float*)&director->
camera_angle);
29 ImGui::DragFloat(
"field of view", &director->
fov);
36 ImGui::Checkbox(
"lock camera", &director->
lock_camera);
37 ImGui::DragFloat(
"camera speed", &director->
speed);
48 ImGui::Checkbox(
"pan-cam", &director->
pancam);
69 if (ImGui::CollapsingHeader(
"internal"))
75 ImGui::DragFloat3(
"camera front", (
float*)&director->
camera_front);
76 ImGui::DragFloat3(
"camera right", (
float*)&director->
camera_right);
BLAM BlamDirector * GetCamera()
Retrieves the director instance.
Definition: director.cpp:24
float fov
The camera's current field of view.
Definition: director.h:67
float far_clip_plane_distance
Definition: director.h:87
BlamVector3 camera_angle
The current looking angle of the camera. X is yaw, Y is pitch, Z is roll (unused atm).
Definition: director.h:61
float zoom_increment_count
The number of steps/increments to use when zooming in and out. Lower numbers will cause the camera to...
Definition: director.h:72
float near_clip_plane_distance
Definition: director.h:86
float vertical_angle
Definition: director.h:84
BlamVector3 camera_right
The calculated point representing the right of the camera.
Definition: director.h:65
bool calculate_angle
Definition: director.h:82
float accel_increment_count
The number of steps/increments to use when accelerating and decelerating. Lower numbers will cause th...
Definition: director.h:78
float horizontal_angle
Definition: director.h:83
float look_sensetivity
The camera's look sensetivity.
Definition: director.h:71
bool move_acceleration
Whether or not camera movements should use acceleration.
Definition: director.h:76
bool show
Controls whether or not the group should be shown. May not be used in all groups.
Definition: debug_ui.h:362
bool auto_verify_coords
Whether or not camera_front and camera_right should be automatically calculated.
Definition: director.h:63
float speed
The camera's current speed.
Definition: director.h:70
Class representing an ImGUI drawing group/draw list item.
Definition: debug_ui.h:359
bool lock_camera
Whether or not the camera is locked. If locked, all keyboard/mouse input is ignored.
Definition: director.h:73
bool look_acceleration
Whether or not camera looking should use acceleration.
Definition: director.h:77
Class representing the Director.
Definition: director.h:19
bool pancam_lock_xy
Whether or not the camera should be locked to X/Y axis when in pan-cam mode.
Definition: director.h:75
bool camera_bouncing
Whether or not the camera should have a bounce/rubber effect when moving and looking around.
Definition: director.h:79
#define ENGINE_TEXT(string_id)
Definition: engine_text.h:7
Legacy namespace to contain data for the legacy ImGUI console.
Definition: debug_ui.h:434
bool pancam
Whether or not the camera is currently in pan-cam mode. Pan-cam locks Z movement of the camera when n...
Definition: director.h:74
@ Text
Master text object that wraps around both BitmapText and DWText.
Definition: render_stack.h:73
BlamVector3 camera_front
The calculated point representing the front of the camera.
Definition: director.h:64
BlamVector3 camera_pos
The current position of the camera.
Definition: director.h:60