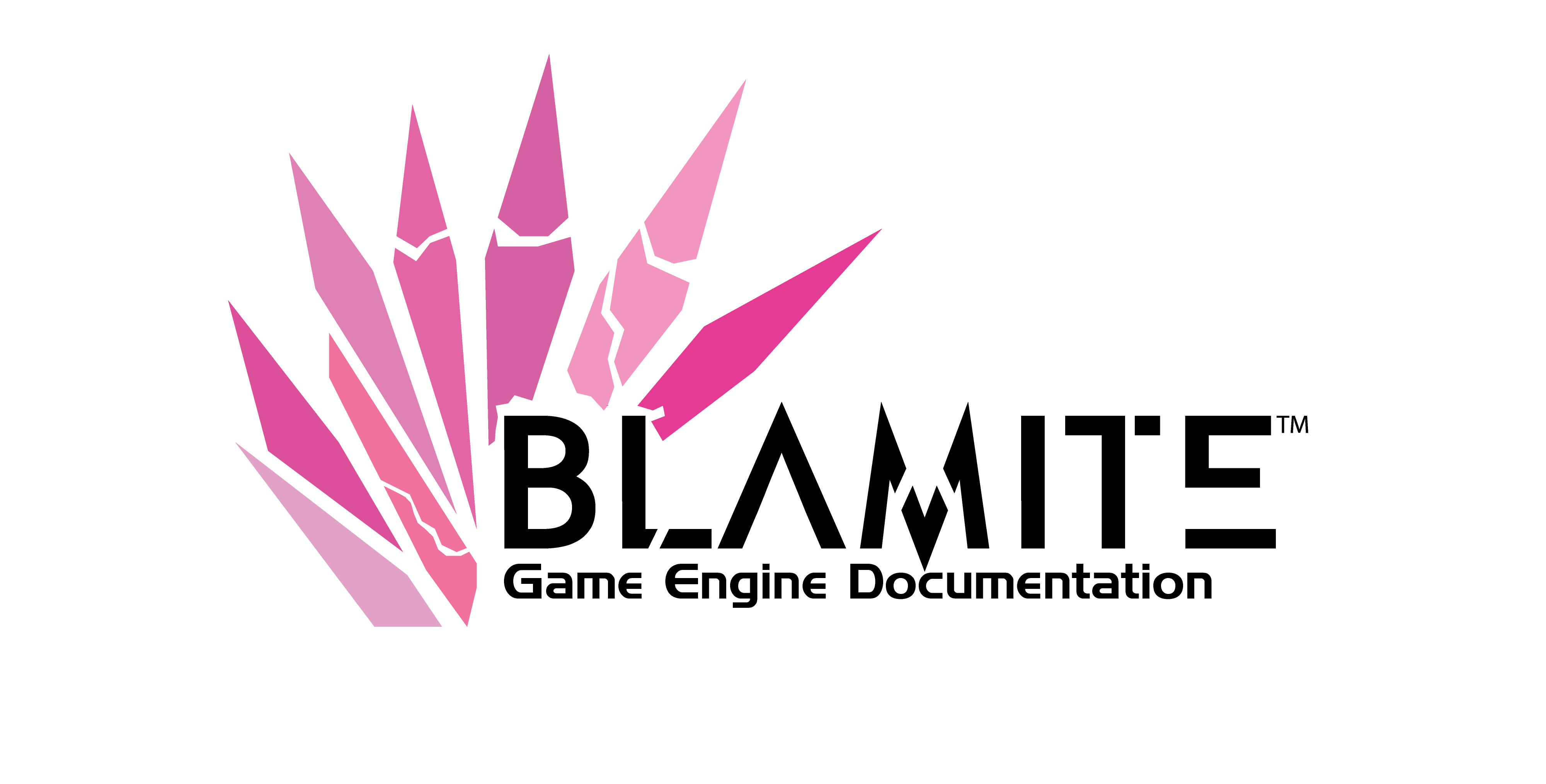 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
69 BLAM HRESULT
DrawRect(
float r,
float g,
float b, D2D1_RECT_F rect,
float thickness);
86 BLAM HRESULT
DWDrawText(std::wstring text, std::wstring font,
float size, D2D1_RECT_F area, D2D1_COLOR_F
color);
104 BLAM HRESULT
DWDrawText(std::wstring text, std::wstring font,
float size,
float left,
float bottom,
float right,
float top,
float r,
float g,
float b);
121 BLAM HRESULT
DWDrawText(std::wstring text, std::wstring font_name,
float size,
int x,
int y, D2D1_COLOR_F
color, IDWriteFontCollection* font_collection,
bool auto_calculate_area, D2D1_RECT_F area);
190 BLAM HRESULT
DrawLine(D2D1_COLOR_F
color, D2D1_POINT_2F start_point, D2D1_POINT_2F end_point,
float thickness);
BLAM HRESULT DrawEllipse(D2D1_COLOR_F outline_color, D2D1_COLOR_F fill_color, D2D1_ELLIPSE ellipse, float thickness, StackItemDrawMode mode)
Draws an ellipse.
Definition: ellipse.cpp:10
int width
Definition: bgfx.cpp:18
BLAM HRESULT DrawBitmapFromResource(int res_id, const char *res_type, D2D1_RECT_F area, int width, int height)
Draws a bitmap pulled from an application resource.
Definition: bitmap.cpp:6
Namespace containing things relating to the Render Stack.
Definition: drawing.h:14
@ color
Definition: render_model.h:12
Namespace containing functions to handle the drawing of Direct2D primitives.
Definition: drawing.h:28
BLAM HRESULT DrawRect(D2D1_COLOR_F outline_color, D2D1_COLOR_F fill_color, D2D1_RECT_F rect, float thickness, StackItemDrawMode mode)
Draws a rectangle.
Definition: rect.cpp:9
#define BLAM
Definition: drawing.h:22
Structure to contain data for a Font.
Definition: fonts.h:68
BLAM HRESULT DrawBitmapText(BlamRendering::RenderStack::BitmapText *text_object, Blam::Content::Fonts::Font *font, bool replace_color_codes)
Draws the specified text using a Bitmap-based Blamite Font.
Definition: text.cpp:550
int height
Definition: bgfx.cpp:19
Class representing text drawn using a Bitmap-based engine font.
Definition: render_stack.h:794
BLAM HRESULT DrawRoundedRect(D2D1_COLOR_F outline_color, D2D1_COLOR_F fill_color, D2D1_ROUNDED_RECT rounded_rect, float thickness, StackItemDrawMode mode)
Draws a rounded rectangle.
Definition: rounded_rect.cpp:10
StackItemDrawMode
Enumerator to specify the stack item draw mode.
Definition: render_stack.h:85
BLAM HRESULT DWDrawText(std::wstring text, std::wstring font, float size, D2D1_RECT_F area, D2D1_COLOR_F color)
Draws a string using DirectWrite.
Definition: dwtext.cpp:16
BLAM HRESULT DrawLine(D2D1_COLOR_F color, D2D1_POINT_2F start_point, D2D1_POINT_2F end_point, float thickness)
Draws a line.
Definition: line.cpp:10