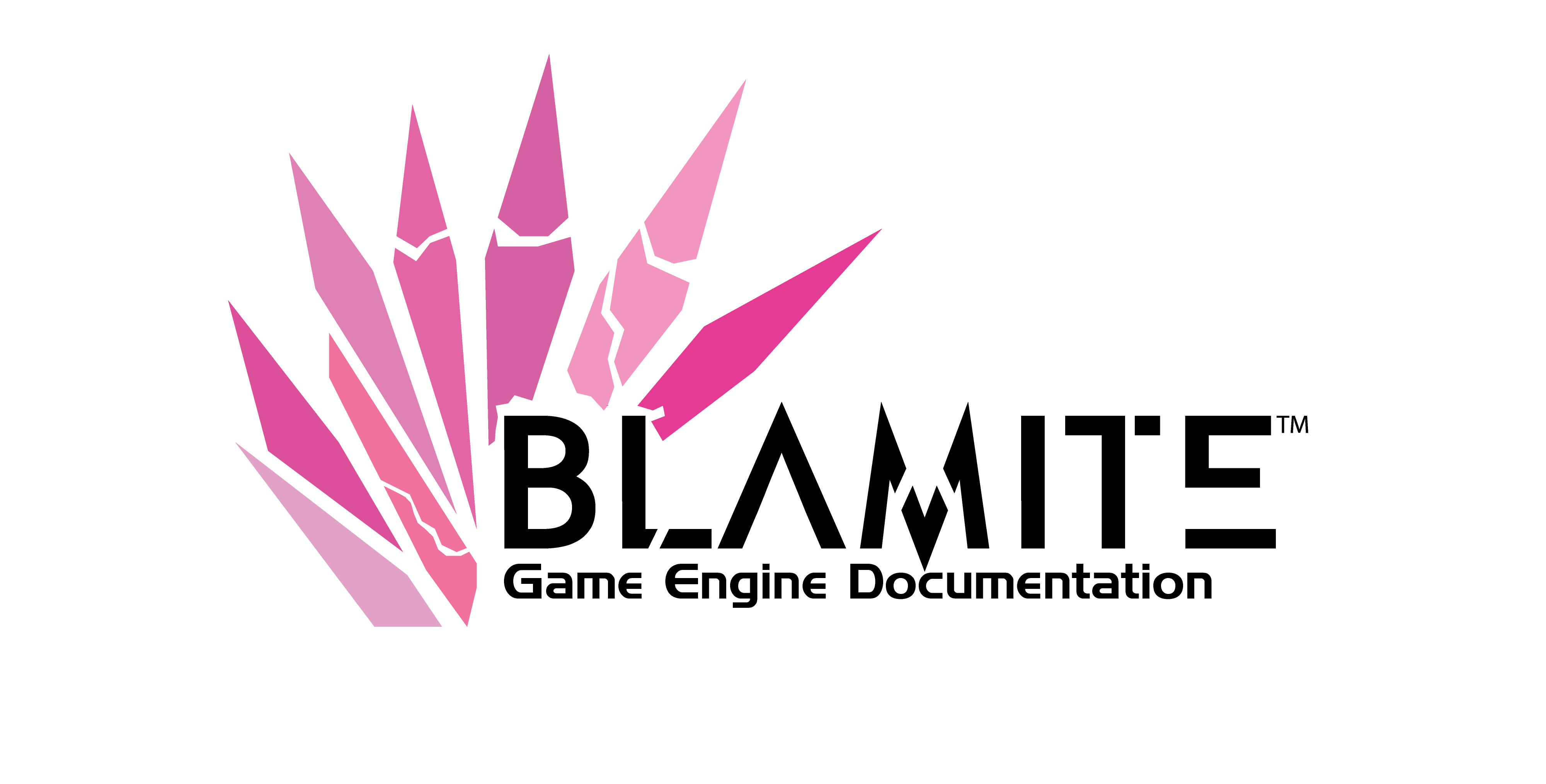 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../../debug_ui.h"
16 bool show_another_window =
false;
17 bool show_test_window =
false;
27 return &show_test_window;
43 static float f = 0.0f;
45 ImGui::SliderFloat(
"float", &f, 0.0f, 1.0f);
56 ImGui::ColorEdit3(
"clear color", (
float*)&clear_col_imgui);
58 BlamColor new_clear_color = BlamColor(clear_col_imgui.x * 255.0f, clear_col_imgui.y * 255.0f, clear_col_imgui.z * 255.0f, clear_col_imgui.w * 255.0f);
63 if (ImGui::Button(
"Test Window")) show_test_window ^= 1;
66 if (ImGui::Button(
"Another Window")) show_another_window ^= 1;
67 if (ImGui::Button(
"Menu Bar")) *
duigvs(
"devtools_bar") ^= 1;
69 ImGui::Text(
"Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
73 if (show_another_window)
75 ImGui::SetNextWindowSize(ImVec2(200, 100));
76 ImGui::Begin(
"Another Window", &show_another_window);
86 ImGui::ShowDemoWindow(&show_test_window);
BLAM ImVec4 * GetClearColor()
Retrieves the current color used to clear the render target.
Definition: render_manage.cpp:613
bool * ShowTestWindow()
Retrieves whether or not the window should be drawn.
Definition: dx11_window.hpp:25
BLAM BlamRenderingEngine GetCurrentRenderingEngine()
Retrieves the current rendering engine being used.
Definition: rendering_abstraction.cpp:103
Class for the default ImGUI DX11 sample window.
Definition: dx11_window.hpp:13
BlamColor * clear_color
Definition: opengl.cpp:21
BLAM BlamColor * GetGlobalAsColor(std::string name)
Retrieves a global's value as a BlamColor.
Definition: globals.cpp:412
bool show
Controls whether or not the group should be shown. May not be used in all groups.
Definition: debug_ui.h:362
void Draw()
Draws the window, as well as the demo window and the "Another Window" if they are enabled.
Definition: dx11_window.hpp:33
Class representing an ImGUI drawing group/draw list item.
Definition: debug_ui.h:359
@ DirectX11
Definition: rendering.h:25
Legacy namespace to contain data for the legacy ImGUI console.
Definition: debug_ui.h:434
@ Text
Master text object that wraps around both BitmapText and DWText.
Definition: render_stack.h:73
BLAM bool * RenderTargetClearing()
Retrieves whether or not to enable render target clearing.
Definition: render_manage.cpp:608
#define duigvs(x)
Macro for Blam::DebugUI::GetVisibility().
Definition: debug_ui.h:19
BLAM GvarUpdateResult UpdateGlobal(std::string name, std::string new_value)
Updates the value of a String global.
Definition: globals.cpp:571