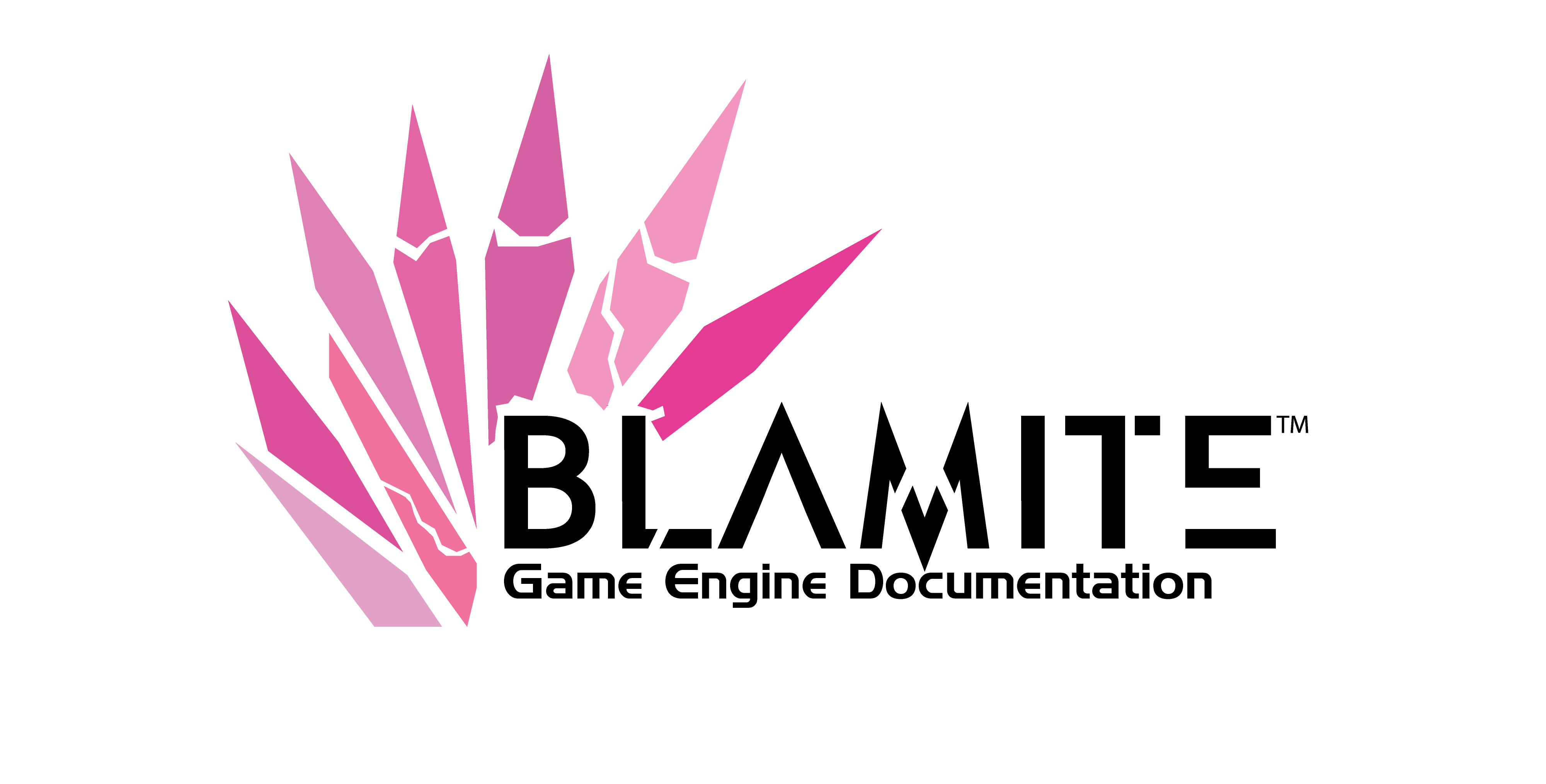 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
8 #include "../../render_stack.h"
10 #include "components/3rdparty/imgui/imgui.h"
13 #include <Strings/components/utils/converters/converters.h>
31 std::thread incremeneter_thread;
32 bool stop_thread =
false;
33 bool thread_stopped =
false;
43 thread_stopped =
true;
58 tick_display->
color = D2D1::ColorF(1, 1, 1, 1);
69 tick_display_2->
color = D2D1::ColorF(1, 1, 1, 1);
79 incremeneter_thread.detach();
86 while (!thread_stopped)
105 std::string new_text = BlamStrings::Converters::BytesToString((
void*)counter,
sizeof(counter));
107 if (new_text.length() < 8)
109 while (new_text.length() != 8)
111 new_text.insert(new_text.begin(),
'0');
115 tick_display->
text = new_text;
116 tick_display_2->
text = new_text;
119 tick_display->
Draw();
120 tick_display_2->
Draw();
BLAM void RegisterListener(BlamEventListener *listener)
Registers a an event listener.
Definition: events.cpp:75
bool visible
Whether or not the object is visible.
Definition: render_stack.h:375
std::string font_id
The ID of the font.
Definition: render_stack.h:844
@ BitmapText
Bitmap-based text, uses Blamite font system.
Definition: render_stack.h:72
StackType type_label
The type of the object.
Definition: render_stack.h:372
BLAM ConfigFile * GetConfig()
Definition: compat.cpp:5
Namespace containing things relating to the Render Stack.
Definition: drawing.h:14
Class to contain the onscreen tick counter display.
Definition: tick_counter.hpp:24
void OnTickEvent(TickEvent *event)
Called when the listener is subscribed to Key Press events, and a new TickEvent is fired.
Definition: tick_counter.hpp:128
std::string text
The text to display.
Definition: render_stack.h:846
D2D1_COLOR_F color
The color of the object.
Definition: render_stack.h:370
Class representing an engine tick event.
Definition: TickEvent.h:10
float y
The Y coordinate of the object.
Definition: render_stack.h:378
#define STACKTYPE_STATS
Definition: render_stack.h:27
void ShowImPropertyEditor()
Shows a set of ImGUI properties associated with the object.
Definition: tick_counter.hpp:123
void Draw()
Draws the stack object.
Definition: BitmapText.cpp:13
void increment()
Definition: tick_counter.hpp:36
bool use_shadow
Whether or not to draw the text with a drop shadow.
Definition: render_stack.h:852
void SetTranslation(float new_x, float new_y)
Sets the translation of the object.
Definition: StackObjectBase.cpp:55
~TickCounter()
Definition: tick_counter.hpp:82
void Subscribe(BlamEventType type)
Subscribes to an event type.
Definition: BlamEventListener.cpp:3
@ EventType_Tick
Definition: BlamEvent.h:9
std::string GetString(std::string option)
Definition: compat.cpp:58
Class representing text drawn using a Bitmap-based engine font.
Definition: render_stack.h:794
Base class for all render stack objects.
Definition: render_stack.h:194
Class representing an Event Listener.
Definition: events.h:27
void Draw()
Draws the stack object.
Definition: tick_counter.hpp:95
TickCounter()
Definition: tick_counter.hpp:46
float x
The X coordinate of the object.
Definition: render_stack.h:377