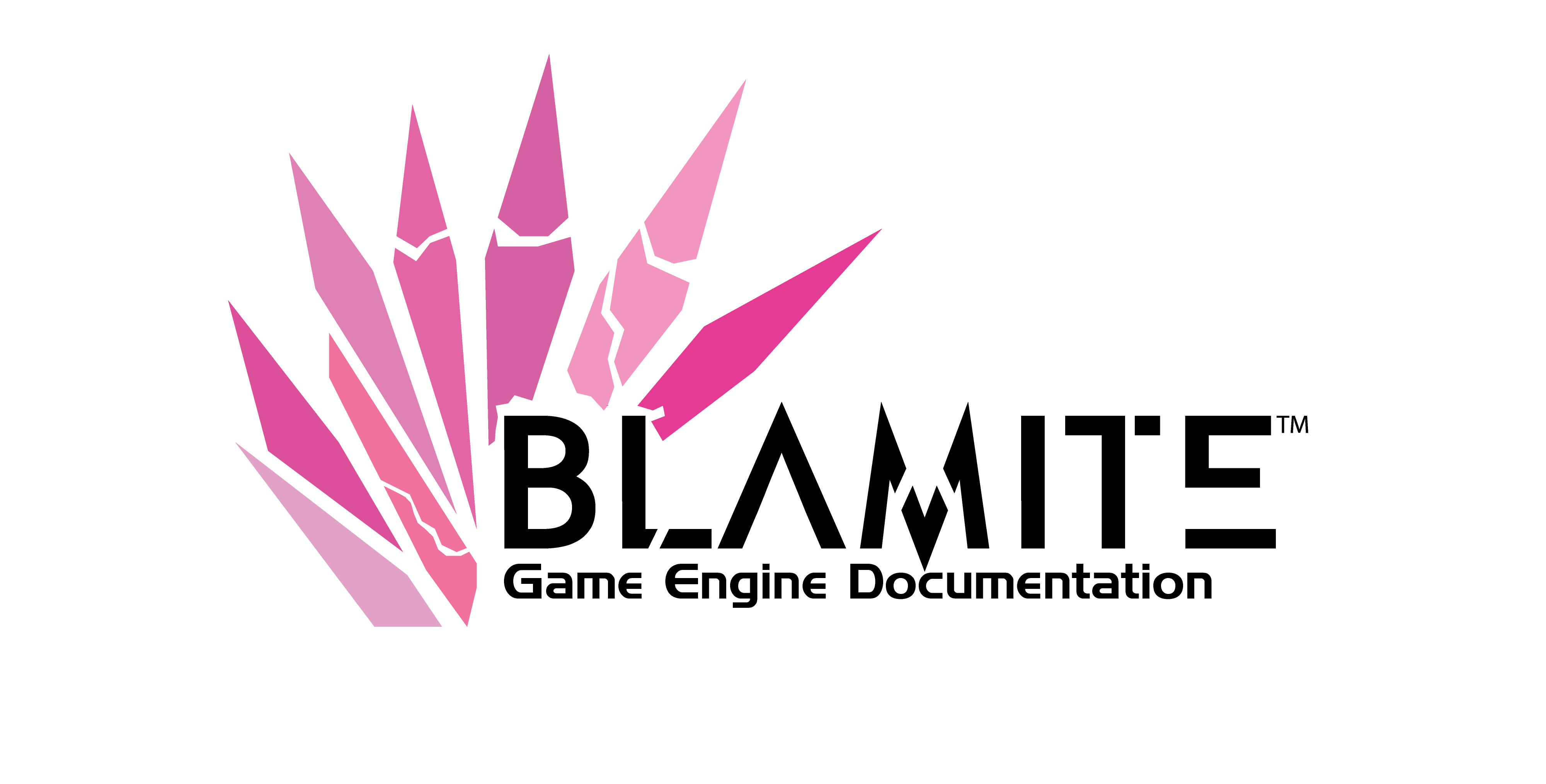 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../../debug_ui.h"
34 int active_index = -1;
37 bool use_raw_values =
false;
38 bool compact_area_input =
false;
40 float shift_limits[2] = { -5, 5 };
43 bool show_unreciognized_popup =
false;
44 bool show_invalid_popup =
false;
45 bool show_outofbounds_popup =
false;
46 bool show_delete_imgui_popup =
false;
57 UnreciognizedTypePopup();
59 IndexOutOfBoundsPopup();
60 DeleteImGuiObjectPopup();
64 if (ImGui::Begin(
"Render Stack Editor", &show))
74 add_item_current_selection = type;
83 if (ImGui::Button(
"Add"))
85 std::string new_object_id =
"item_" +
GetStack()->size();
89 show_invalid_popup =
true;
93 show_invalid_popup =
true;
145 show_unreciognized_popup =
true;
150 if (ImGui::Button(
"Remove"))
152 if (
GetStack()->at(active_index) !=
nullptr)
156 show_delete_imgui_popup =
true;
165 show_outofbounds_popup =
true;
170 if (ImGui::Button(
"Duplicate"))
180 ImGui::SetColumnWidth(0, 200);
182 if (ImGui::Button(
"^"))
189 if (ImGui::Button(
"v"))
194 if (ImGui::ListBoxHeader(
"", ImVec2(ImGui::GetColumnWidth() - 15, -1)))
196 for (
int i = 0; i <
GetStack()->size(); i++)
200 std::string label = std::to_string(i) +
": (" + obj->
GetType() +
")";
202 const char* label_cstr = label.c_str();
206 if (i == active_index)
211 if (ImGui::Selectable(label_cstr, active))
216 if (ImGui::IsItemHovered())
218 ImGui::BeginTooltip();
219 ImGui::PushTextWrapPos(450.0f);
223 std::string object_index =
"index: " + std::to_string(i);
224 std::string object_type =
"type : " + obj->
GetType();
236 ImGui::PopTextWrapPos();
241 ImGui::ListBoxFooter();
246 if (active_object !=
nullptr)
268 active_index = index;
277 std::string header = std::to_string(active_index) +
": (" + active_object->
GetType() +
")";
281 ImGui::Checkbox(
"use raw values", &use_raw_values);
284 float object_width = active_object->
area.right - active_object->
area.left;
285 float object_height = active_object->
area.bottom - active_object->
area.top;
287 if (object_width < 0 || object_height < 0)
289 ImGui::TextColored(ImVec4(255, 0, 0, 255),
"[warn]");
290 if (ImGui::IsItemHovered())
292 ImGui::BeginTooltip();
293 ImGui::PushTextWrapPos(450.0f);
294 ImGui::Text(
"height and/or width will be negative on conversion!");
296 if (object_width < 0)
298 std::string width_msg =
"object width would be less than 0 (" + std::to_string(object_width) +
")";
302 if (object_height < 0)
304 std::string height_msg =
"object height would be less than 0 (" + std::to_string(object_height) +
")";
308 ImGui::PopTextWrapPos();
314 ImGui::TextColored(ImVec4(0, 255, 0, 255),
"[ok]");
317 Blam::DebugUI::Widgets::ShowHelpMarker(
"when enabled, exposes the raw values seen by directx\r\nwhen disabled, friendlier fields are displayed instead\r\n\r\nthe status indicator shows either [ok] or [warn] based on what the object height will be upon disabling real rect sizes");
319 if (ImGui::BeginTabBar(
"rse_tabs", ImGuiTabBarFlags_NoTooltip))
321 if (ImGui::BeginTabItem(
"Information"))
325 std::string item_type =
"object type: " + active_object->
GetType();
326 std::string item_index =
"stack index: " + std::to_string(active_index);
327 std::string unique_id =
"unique id: " + active_object->
unique_id;
339 std::string unique_props_label =
"Properties for " + active_object->
GetType();
342 if (ImGui::BeginTabItem(unique_props_label.c_str()))
361 if (ImGui::BeginTabItem(
"Base Properties"))
367 ImGui::Checkbox(
"compact area input", &compact_area_input);
369 if (compact_area_input)
371 ImGui::DragFloat4(
"area", (
float*)&active_object->
area);
375 ImGui::DragFloat(
"area left", &active_object->
area.left);
376 ImGui::DragFloat(
"area top", &active_object->
area.top);
377 ImGui::DragFloat(
"area right", &active_object->
area.right);
378 ImGui::DragFloat(
"area bottom", &active_object->
area.bottom);
381 std::string value_helper =
"top: " + std::to_string(active_object->
area.top)
382 +
"\r\nbottom: " + std::to_string(active_object->
area.bottom)
383 +
"\r\nleft: " + std::to_string(active_object->
area.left)
384 +
"\r\nright: " + std::to_string(active_object->
area.right);
390 ImGui::DragFloat(
"width", &active_object->
width, 1, 0, 2147483647,
"width: %.3f");
391 ImGui::DragFloat(
"height", &active_object->
height, 1, 0, 2147483647,
"height: %.3f");
397 ImGui::ColorEdit4(
"color", (
float*)&active_object->
color);
398 ImGui::SliderInt(
"z order", &active_object->
z_order, 0, 255);
403 if (ImGui::CollapsingHeader(
"shifting"))
410 float rect_shift[2] = { 0, 0 };
411 ImGui::SliderFloat2(
"shift", rect_shift, shift_limits[0], shift_limits[1]);
413 ImGui::InputFloat2(
"shift bounds", shift_limits);
421 if ((active_object->
area.top > 0 || rect_shift[0] > 0) && (active_object->
area.bottom < target_size.height || rect_shift[0] < 0))
423 active_object->
area.top = active_object->
area.top + rect_shift[0];
424 active_object->
area.bottom = active_object->
area.bottom + rect_shift[0];
427 if ((active_object->
area.left > 0 || rect_shift[1] > 0) && (active_object->
area.right < target_size.width || rect_shift[1] < 0))
429 active_object->
area.left = active_object->
area.left + rect_shift[1];
430 active_object->
area.right = active_object->
area.right + rect_shift[1];
435 active_object->
area.top = active_object->
area.top + rect_shift[0];
436 active_object->
area.bottom = active_object->
area.bottom + rect_shift[0];
437 active_object->
area.left = active_object->
area.left + rect_shift[1];
438 active_object->
area.right = active_object->
area.right + rect_shift[1];
445 if (ImGui::CollapsingHeader(
"translation"))
453 float x = active_object->
area.left;
454 float y = active_object->
area.top;
456 float x_min = -2147483647;
457 float x_max = 2147483647;
459 float y_min = -2147483647;
460 float y_max = 2147483647;
465 x_max = target_size.width - active_object->
width;
468 y_max = target_size.height - active_object->
height;
475 ImGui::DragFloat(
"x coord", &active_object->
x,
translate_speed, x_min, x_max,
"x: %.3f");
476 ImGui::DragFloat(
"y coord", &active_object->
y,
translate_speed, y_min, y_max,
"y: %.3f");
522 #pragma region dialogs
529 if (show_delete_imgui_popup)
531 ImGui::OpenPopup(
"WARNING");
532 if (ImGui::BeginPopupModal(
"WARNING", &show_delete_imgui_popup, ImGuiWindowFlags_NoResize))
534 ImGui::Text(
"you are trying to delete imgui stack object! this will remove imgui entirely, including stack editor!");
537 ImGui::Text(
"tip: you can re-add by enabling the win32 menu bar and choosing Test > Restore ImGUI");
539 if (ImGui::Button(
"yes"))
542 show_delete_imgui_popup =
false;
545 if (ImGui::Button(
"no"))
547 show_delete_imgui_popup =
false;
563 if (show_outofbounds_popup)
565 ImGui::OpenPopup(
"index out of bounds");
566 if (ImGui::BeginPopupModal(
"index out of bounds", &show_outofbounds_popup, ImGuiWindowFlags_NoResize))
568 ImGui::Text(
"selected render stack item index was out of bounds (most likely no object was selected)");
571 std::string selected_index_text =
"selected item index: " + active_index;
575 if (ImGui::Button(
"ok"))
577 show_outofbounds_popup =
false;
593 if (show_unreciognized_popup)
595 ImGui::OpenPopup(
"unreciognized or invalid type");
596 if (ImGui::BeginPopupModal(
"unreciognized or invalid type", &show_unreciognized_popup, ImGuiWindowFlags_NoResize))
598 ImGui::Text(
"invalid type specified, unreciognized type,\r\nor no object add functionality implemented");
600 ImGui::Text(
"hint: look for 'render_stack_editor.cpp' and\r\ncheck there");
602 if (ImGui::Button(
"ok"))
604 show_unreciognized_popup =
false;
620 if (show_invalid_popup)
622 ImGui::OpenPopup(
"invalid type");
623 if (ImGui::BeginPopupModal(
"invalid type", &show_invalid_popup, ImGuiWindowFlags_NoResize))
628 ImGui::BulletText(
"imgui items can only be added once - as that object controls\r\nthe entirety of imgui - you can't have two of them");
629 ImGui::BulletText(
"generic stack objects have no drawing functionality and thus\r\nwould do nothing but waste memory");
631 ImGui::Text(
"if you tried to add another object type, then the functionality\r\nwas either deliberately disabled or something got borked - check\r\n'render_stack_editor.hpp' for clues");
634 if (ImGui::Button(
"sure thing bro"))
636 show_invalid_popup =
false;
Class containing data for a text object using DirectWrite.
Definition: render_stack.h:656
bool rect_limit_to_window
Definition: Line.cpp:10
BLAM std::vector< StackType > GetStackTypesList()
Retrieves a list of all available stack types.
Definition: render_stack.cpp:191
BLAM std::vector< StackObjectBase * > * GetStack()
Retrieves the render stack contents.
Definition: render_stack.cpp:65
void UpdateAllProperties()
Applies any modified parent properties to the appropriate child object.
Definition: Text.cpp:74
void PropertyView()
Shows the property view controls, base controls, and any controls specific to the active render stack...
Definition: render_stack_editor.hpp:275
#define STACKTYPE_ROUNDED_RECT
Definition: render_stack.h:16
#define STACKTYPE_DEBUG_MENU
Definition: render_stack.h:28
#define STACKTYPE_DWTEXT
Definition: render_stack.h:17
std::string GetType()
Retrieves the type of item that this stack object is.
Definition: StackObjectBase.cpp:125
@ BitmapText
Bitmap-based text, uses Blamite font system.
Definition: render_stack.h:72
void RefreshTranslation()
Refreshes any translation data and applies them to the child objects.
Definition: Text.cpp:55
BLAM ID2D1DeviceContext * GetD2DRenderTarget()
Retrieves the Direct2D render target.
Definition: render_manage.cpp:598
StackType type_label
The type of the object.
Definition: render_stack.h:372
void DeleteImGuiObjectPopup()
Shows a confirmation dialog when trying to delete the ImGUI stack object.
Definition: render_stack_editor.hpp:527
#define STACKTYPE_RECT
Definition: render_stack.h:15
@ Generic
Generic stack type - should probably not be used.
Definition: render_stack.h:57
Namespace containing things relating to the Render Stack.
Definition: drawing.h:14
void IndexOutOfBoundsPopup()
Shows a warning dialog indicating that the selected render stack index was out of bounds.
Definition: render_stack_editor.hpp:561
Class containing data for a Rectangle object.
Definition: render_stack.h:418
int z_order
The Z-Order of the object.
Definition: render_stack.h:371
BLAM void LogEventForce(std::string message, BlamLogLevel severity)
Forcibly logs a message to the log and/or console.
Definition: aliases.cpp:218
D2D1_COLOR_F color
The color of the object.
Definition: render_stack.h:370
Class containing data for a Rounded Rectangle object.
Definition: render_stack.h:463
float y
The Y coordinate of the object.
Definition: render_stack.h:378
Class containing data for a Bitmap object.
Definition: render_stack.h:573
Class containing data for an Ellipse object.
Definition: render_stack.h:521
void BasePropertiesTab()
Shows properties that are part of BlamRendering::RenderStack::StackObjectBase.
Definition: render_stack_editor.hpp:359
@ Line
Direct2D Line.
Definition: render_stack.h:65
void PokeSize()
Updates the area of the object to account for any width/height changes.
Definition: StackObjectBase.cpp:119
void Draw()
Draws the render stack editor dialog, as well as any active popups if need be.
Definition: render_stack_editor.hpp:54
#define STACKTYPE_ELLIPSE
Definition: render_stack.h:18
#define WSV_NONE
Macro for 'None' log seveirty. Original pre-enum value was 0.
Definition: logger.h:16
#define STACKTYPE_LINE
Definition: render_stack.h:19
Class representing an ImGUI drawing group/draw list item.
Definition: debug_ui.h:359
void InvalidTypePopup()
Shows a warning dialog indicating that the selected render stack type is invalid.
Definition: render_stack_editor.hpp:618
#define STACKTYPE_TEXT
Definition: render_stack.h:29
void SelectItem(int index, StackObjectBase *obj)
Changes the selected item and stack object.
Definition: render_stack_editor.hpp:266
#define STACKTYPE_BITMAP
Definition: render_stack.h:20
std::string unique_id
The unique ID of this object.
Definition: render_stack.h:382
void UnreciognizedTypePopup()
Shows a warning dialog indicating that the user tried to add a new unknown stack type to the render s...
Definition: render_stack_editor.hpp:591
void PokeTranslation()
Updates the area of the object to account for any x/y coordinate changes.
Definition: StackObjectBase.cpp:97
#define STACKTYPE_BITMAP_TEXT
Definition: render_stack.h:24
D2D1_RECT_F area
The area of the object.
Definition: render_stack.h:369
BLAM int AddToStack(std::string id, StackObjectBase *object)
Adds an item to the render stack.
Definition: render_stack.cpp:12
float width
The width of the object.
Definition: render_stack.h:379
virtual void ShowImPropertyEditor()
Shows a set of ImGUI properties associated with the object.
Definition: render_stack.h:239
Class used to wrap around BitmapText and DWText, making the usage of both of them directly unnecessar...
Definition: render_stack.h:896
BLAM void RemoveFromStack(std::string id)
Removes an item from the render stack.
Definition: render_stack.cpp:26
BLAM std::string GetStackTypeLabel(StackType type)
Retrieves a string representation of the specified stack type.
Definition: render_stack.cpp:133
@ Bitmap
Direct2D Bitmap.
Definition: render_stack.h:66
float height
The height of the object.
Definition: render_stack.h:380
#define STACKTYPE_GENERIC
Definition: render_stack.h:13
void PokeEllipseTranslation()
Updates the ellipse origin point based on the x/y coordinates.
Definition: ellipse.cpp:23
Class representing text drawn using a Bitmap-based engine font.
Definition: render_stack.h:794
Base class for all render stack objects.
Definition: render_stack.h:194
Legacy namespace to contain data for the legacy ImGUI console.
Definition: debug_ui.h:434
void PokeRoundedRectArea()
Updates the area of the rounded rectangle after area modification.
Definition: RoundedRectangle.cpp:23
@ Text
Master text object that wraps around both BitmapText and DWText.
Definition: render_stack.h:73
StackType
Enumerator to determine the type of render stack item.
Definition: render_stack.h:54
@ DWText
DirectWrite Text, does not utilize our font system - probably dont use this.
Definition: render_stack.h:63
#define STACKTYPE_IMGUI
Definition: render_stack.h:14
Class for the Render Stack Editor.
Definition: render_stack_editor.hpp:31
float translate_speed
Definition: Line.cpp:11
float x
The X coordinate of the object.
Definition: render_stack.h:377
void PokeLineTranslation()
Pokes the line start/end points to match the coordinates/area.
Definition: Line.cpp:37
Class containing data for a Line object.
Definition: render_stack.h:599