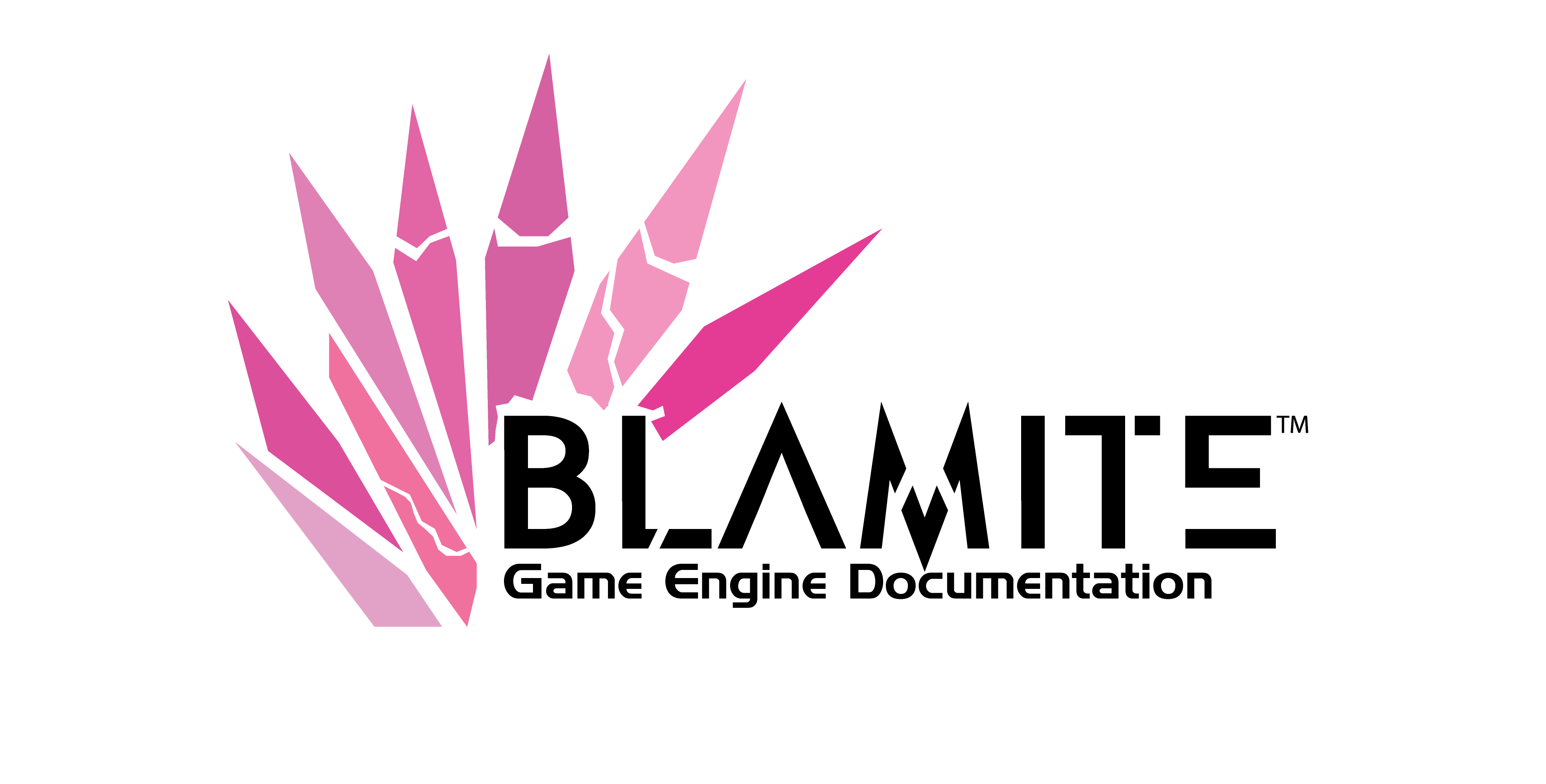 |
Blamite Game Engine - blam!
00346.12.11.21.0529.blamite
The core library for the Blamite Game Engine.
|
IMGUI_API bool InputText(const char *label, char *buf, size_t buf_size, ImGuiInputTextFlags flags=0, ImGuiInputTextCallback callback=NULL, void *user_data=NULL)
Definition: imgui_widgets.cpp:3068
#define IMGUI_API
Definition: imgui.h:57
int ImGuiInputTextFlags
Definition: imgui.h:150
Add a fourth parameter to bake specific font ranges NULL
Definition: README.txt:57
Definition: imgui_extensions.h:5
IMGUI_API bool InputTextWithHint(const char *label, const char *hint, char *buf, size_t buf_size, ImGuiInputTextFlags flags=0, ImGuiInputTextCallback callback=NULL, void *user_data=NULL)
Definition: imgui_widgets.cpp:3079
IMGUI_API bool InputTextMultiline(const char *label, char *buf, size_t buf_size, const ImVec2 &size=ImVec2(0, 0), ImGuiInputTextFlags flags=0, ImGuiInputTextCallback callback=NULL, void *user_data=NULL)
Definition: imgui_widgets.cpp:3074
int(* ImGuiInputTextCallback)(ImGuiInputTextCallbackData *data)
Definition: imgui.h:156