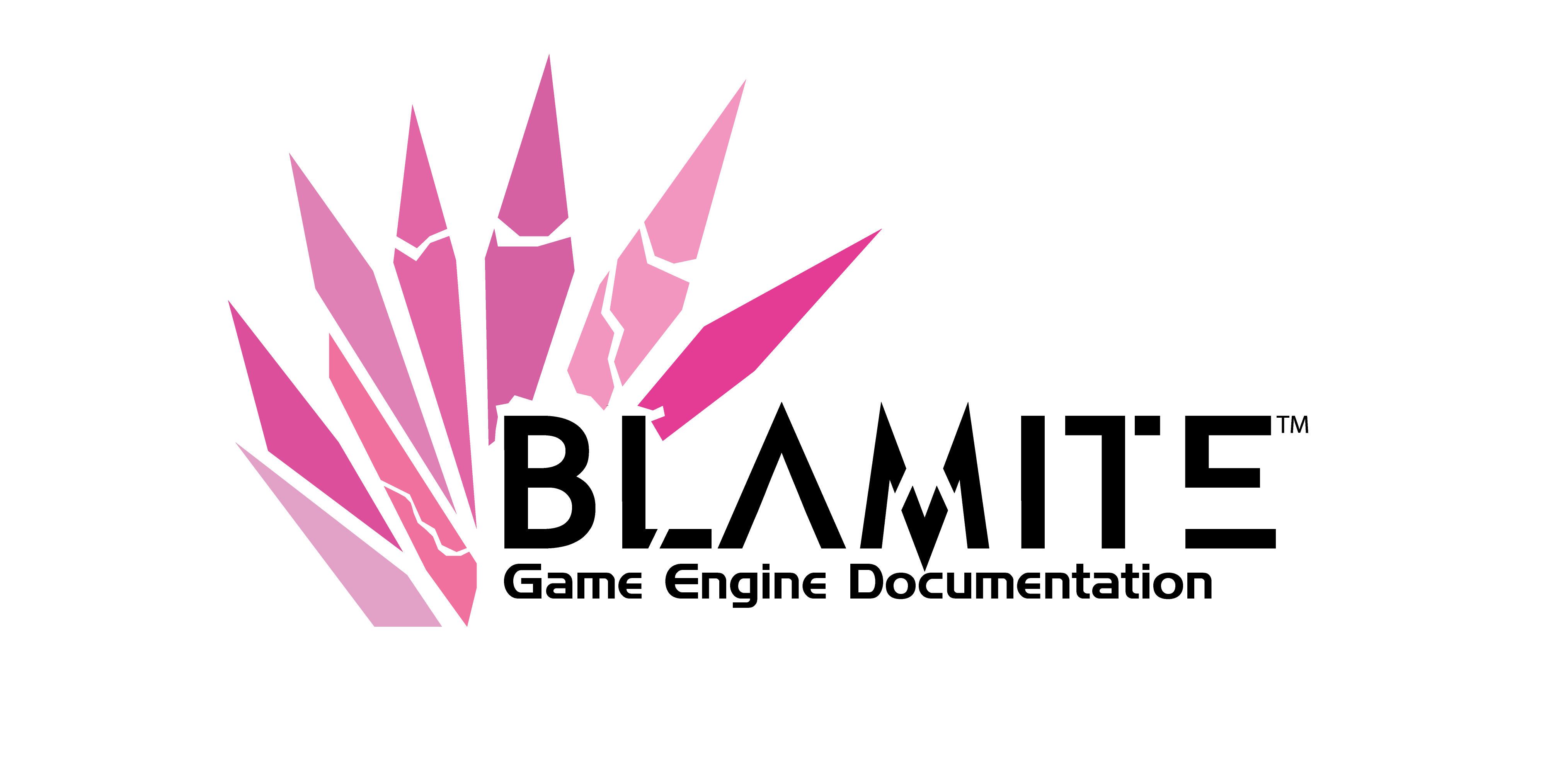 |
Blamite Game Engine - Guerilla (Library)
00359.07.01.22.2202.blamite
The tag editor for the Blamite Game Engine.
|
Go to the documentation of this file.
6 #ifndef GUERILLA_LIB_INTERNAL
7 #define GUERILLA_LIB_INTERNAL
10 #ifdef GUERILLA_LIB_EXPORTS
11 #define GUERILLA_LIB_API __declspec(dllexport)
13 #define GUERILLA_LIB_API __declspec(dllimport)
144 std::string display_name =
"";
145 std::string description =
"";
146 std::string field_id =
"";
227 std::string field_id =
"";
242 virtual std::string GenerateXMLString();
249 virtual std::vector<char> GetValueAsBytes();
277 bool HasPluginField();
Class representing a tag field.
Definition: fields.h:219
@ Int16
Indicates that the field is a 16-bit integer.
bool flag6
Definition: fields.h:66
bool flag1
Definition: fields.h:61
bool flag13
Definition: fields.h:74
bool flag4
Definition: fields.h:64
bool flag31
Definition: fields.h:94
bool flag0
Definition: fields.h:36
@ Int32
Indicates that the field is a 32-bit integer.
bool flag5
Definition: fields.h:26
bool flag11
Definition: fields.h:72
bool flag8
Definition: fields.h:69
@ Enum8
Indicates that the field is an Enum8.
bool flag19
Definition: fields.h:81
bool flag27
Definition: fields.h:90
bool flag0
Definition: fields.h:60
Typedef for a bitfield32 field, used in tag data definitions.
Definition: fields.h:58
bool flag7
Definition: fields.h:67
@ Block
Indicates that the field is a tag block.
bool flag12
Definition: fields.h:49
bool flag15
Definition: fields.h:76
bool flag8
Definition: fields.h:45
bool flag14
Definition: fields.h:51
bool flag9
Definition: fields.h:70
@ Ascii
Indicates that the field is a string of text.
bool flag2
Definition: fields.h:23
bool flag29
Definition: fields.h:92
@ Dataref
Indicates that the field is a data reference.
@ Enum16
Indicates that the field is an Enum16.
bool flag5
Definition: fields.h:41
bool flag11
Definition: fields.h:48
bool flag28
Definition: fields.h:91
@ Bitfield16
Indicates that the field is a Bitfield16.
bool flag3
Definition: fields.h:39
BlamTagFieldType
Enumerator containing all possible tag field types.
Definition: fields.h:103
@ Int8
Indicates that the field is an 8-bit integer.
bool flag17
Definition: fields.h:79
bool flag9
Definition: fields.h:46
bool flag30
Definition: fields.h:93
bool flag26
Definition: fields.h:89
Typedef for a bitfield8 field, used in tag data definitions.
Definition: fields.h:19
@ Comment
Indicates that the field is a comment. These do not store any tag data.
bool flag18
Definition: fields.h:80
bool flag13
Definition: fields.h:50
bool flag25
Definition: fields.h:88
@ Float32
Indicates that the field is a Float32.
bool flag6
Definition: fields.h:27
Class representing a plugin field.
Definition: fields.h:134
bool flag3
Definition: fields.h:63
Class representing a Tag.
Definition: tags.h:126
bool flag7
Definition: fields.h:28
bool flag24
Definition: fields.h:87
bool flag16
Definition: fields.h:78
bool flag20
Definition: fields.h:82
bool flag4
Definition: fields.h:25
@ Tagref
Indicates that the field is a tag reference.
bool flag21
Definition: fields.h:83
bool flag2
Definition: fields.h:62
bool flag1
Definition: fields.h:22
bool flag7
Definition: fields.h:43
Class representing a Plugin.
Definition: tags.h:35
bool flag15
Definition: fields.h:52
@ Bitfield8
Indicates that the field is a Bitfield8.
Typedef for a bitfield16 field, used in tag data definitions.
Definition: fields.h:34
bool flag6
Definition: fields.h:42
bool flag1
Definition: fields.h:37
bool flag10
Definition: fields.h:47
bool flag5
Definition: fields.h:65
bool flag14
Definition: fields.h:75
bool flag0
Definition: fields.h:21
@ Unspecified
Indicates that the field's type is not specified. Fields with this type should be considered invalid.
bool flag3
Definition: fields.h:24
@ StringId
Indicates that the field is a String ID.
bool flag4
Definition: fields.h:40
bool flag12
Definition: fields.h:73
bool flag22
Definition: fields.h:84
@ Bitfield32
Indicates that the field is a Bitfield32.
#define GUERILLA_LIB_API
Definition: fields.h:13
bool flag23
Definition: fields.h:85
@ Enum32
Indicates that the field is an Enum32.
bool flag2
Definition: fields.h:38
bool flag10
Definition: fields.h:71