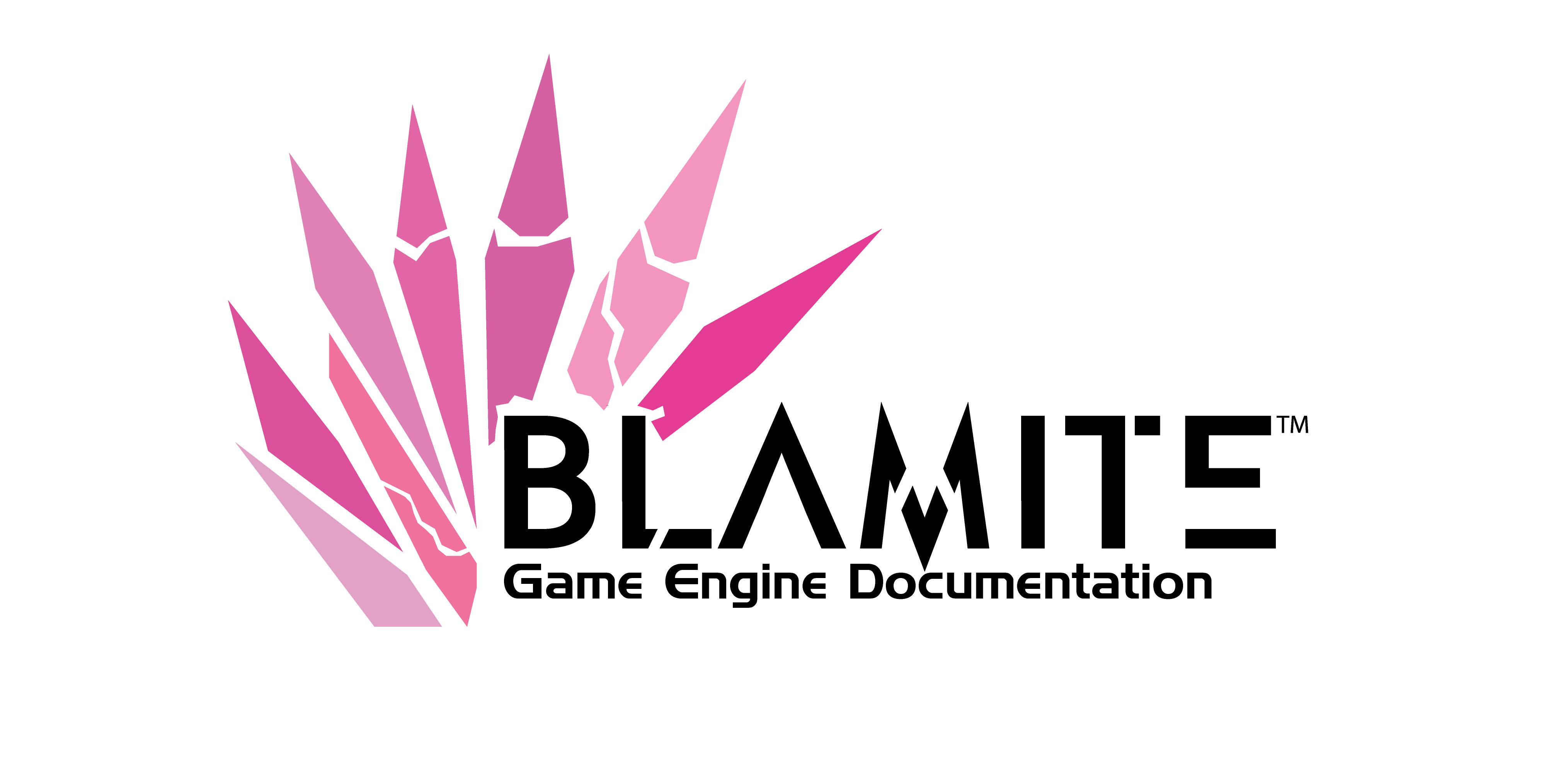 |
Blamite Game Engine - Keystone
00390.07.02.23.1947.blamite
A library that enables the use of Qt in Blamite's editing tools.
|
Go to the documentation of this file.
6 #if QT_VERSION < QT_VERSION_CHECK(5, 14, 0)
31 #if QT_VERSION < QT_VERSION_CHECK(5, 14, 0)
44 QString
comments(quint64 line,
int column)
const;
47 void clear(quint64 line);
54 void metadata(qint64 begin, qint64 end,
const QColor &fgcolor,
const QColor &bgcolor,
const QString &
comment);
57 void metadata(quint64 line,
int start,
int length,
const QColor& fgcolor,
const QColor& bgcolor,
const QString&
comment);
58 void color(quint64 line,
int start,
int length,
const QColor& fgcolor,
const QColor& bgcolor);
59 void foreground(quint64 line,
int start,
int length,
const QColor& fgcolor);
60 void background(quint64 line,
int start,
int length,
const QColor& bgcolor);
61 void comment(quint64 line,
int start,
int length,
const QString &
comment);
73 QHash<quint64, QHexLineMetadata> m_metadata;
74 QVector<QHexMetadataAbsoluteItem> m_absoluteMetadata;
77 #endif // QHEXMETADATA_H