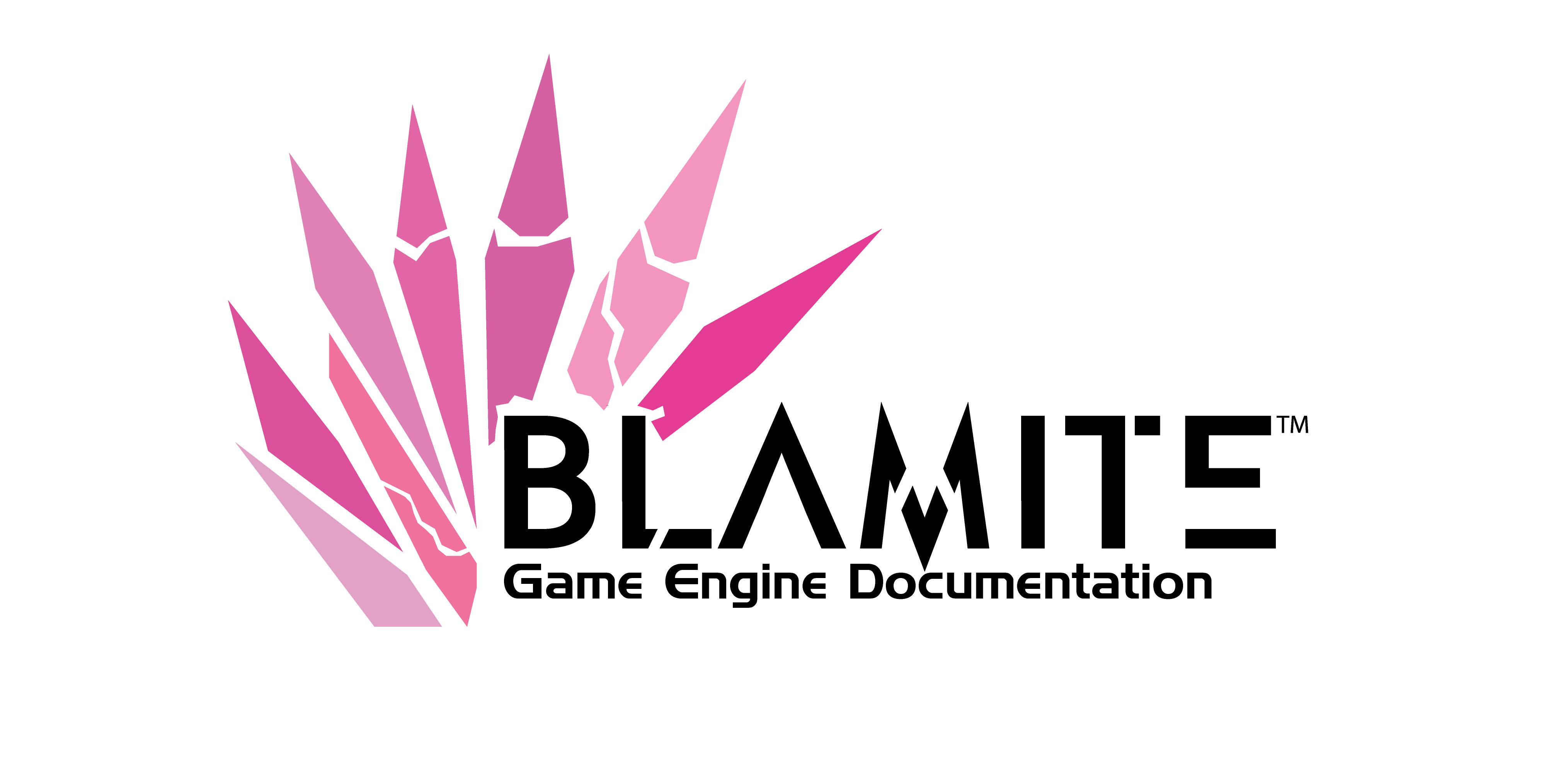 |
Blamite Game Engine - Keystone
00390.07.02.23.1947.blamite
A library that enables the use of Qt in Blamite's editing tools.
|
Go to the documentation of this file.
4 #include "ui_qt_message_box.h"
44 void button_Clicked(QAbstractButton* button);
47 Ui::qt_message_box ui;
49 QGraphicsScene* scene;
50 QGraphicsPixmapItem* pixmap_item;
51 std::string icon_resource_name;
55 void resizeEvent(QResizeEvent* event);
56 void showEvent(QShowEvent* event);
61 void UpdateIconView();
@ Default_NotSet
The dialog result has not yet been set.
KeystoneDialogResult
Enumerator containing all possible generic dialog results.
Definition: keystone_api.h:30
@ AbortRetryIgnore
Dialog is shown with an [Abort], [Retry] and [Ignore] button.
KeystoneMessageBoxButtons
Enumerator containing different possible button combinations.
Definition: qt_message_box.h:23
@ Ok
Dialog is shown with an [OK] button.
@ Error
Dialog icon will be a red circle with an X.
@ Cancel
The user pressed the 'Cancel' button.
qt_message_box(KeystoneMessageBoxType type, KeystoneMessageBoxButtons buttons, QString title, QString message, QWidget *parent=Q_NULLPTR)
Initializes a new message box.
Definition: qt_message_box.cpp:7
@ OK
The user pressed the 'OK' button.
KeystoneMessageBoxType
Enumerator containing different possible message box types, primarily used to control the icon that i...
Definition: qt_message_box.h:10
@ Ignore
The user pressed the 'Ignore' button.
@ YesNo
Dialog is shown with a [Yes] and [No] button.
@ YesNoCancel
Dialog is shown with a [Yes], [No], and [Cancel] button.
@ Warning
Dialog icon will be a yellow triangle with an exclamation mark.
KEYSTONE BlamEditorTheme * GetActiveTheme()
Retrieves the currently selected theme.
Definition: themes.cpp:335
@ Yes
The user pressed the 'Yes' button.
@ Help
Dialog icon will be a purple book with a question mark.
~qt_message_box()
Definition: qt_message_box.cpp:104
@ None
The dialog will not contain an icon.
KeystoneDialogResult GetResult()
Retrieves the dialog result.
Definition: qt_message_box.cpp:167
@ Question
Dialog icon will be a speech bubble with a question mark.
@ Abort
The user pressed the 'Abort' button.
@ No
The user pressed the 'No' button.
@ OkCancel
Dialog is shown with an [OK] and [Cancel] button.
@ Info
Dialog icon will be a white circle with an i.
@ Retry
The user pressed the 'Retry' button.
A basic message box dialog.
Definition: qt_message_box.h:39