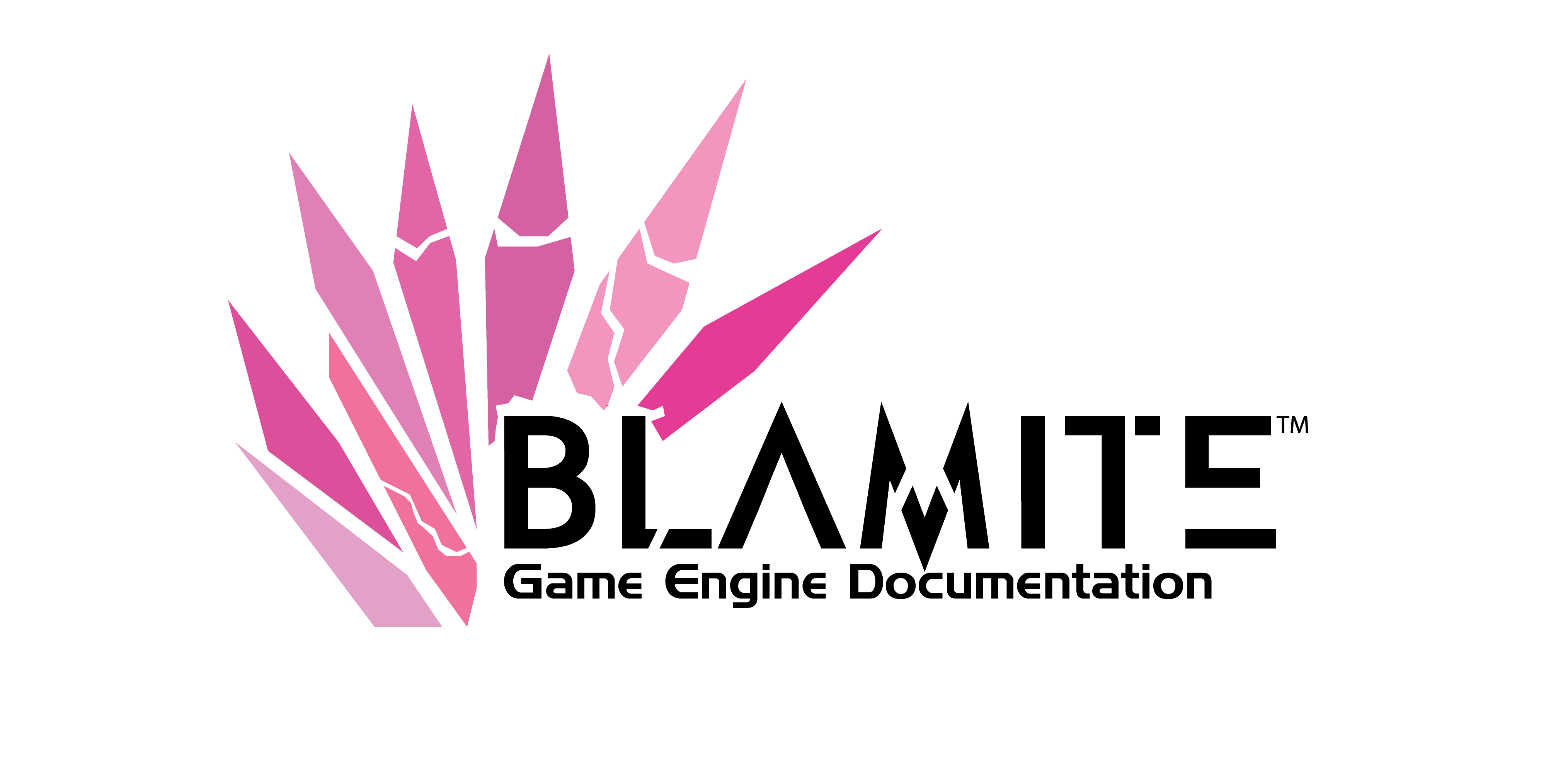 |
Blamite Game Engine - Guerilla (Library)
00402.09.29.23.0627.blamite
The tag editor for the Blamite Game Engine.
|
Go to the documentation of this file.
7 #ifdef GUERILLA_LIB_EXPORTS
8 #define GUERILLA_LIB_API __declspec(dllexport)
10 #define GUERILLA_LIB_API __declspec(dllimport)
49 void ParseCommentType(std::string comment_type_string);
52 std::string
GenerateCppStructString(std::string line_prefix =
"",
bool generate_doxygen_comments =
true)
override;
53 std::string
GenerateCppStructString(std::string line_prefix =
"",
bool generate_doxygen_comments =
true,
bool include_description =
false);
55 bool ParseXMLData(rapidxml::xml_node<>* field_node)
override;
Class representing a tag field.
Definition: fields.h:231
virtual BlamTagField * GenerateTagField(BlamTag *tag)
Generates a new tag field from this plugin field.
Definition: BlamPluginField.cpp:41
virtual std::string GenerateXMLString()
Generates a string representing this plugin field.
Definition: BlamPluginField.cpp:61
std::string field_id
The ID of this field.
Definition: fields.h:243
BlamTagFieldType GetType()
Retrieves the type of this field.
Definition: BlamPluginField.cpp:31
virtual std::string GenerateXMLString()
Generates a string with the field ID and value, ready to be written to an XML file.
Definition: BlamTagField.cpp:9
std::string display_name
The display name of the field.
Definition: fields.h:81
Class representing a plugin field.
Definition: fields.h:71
Class representing a Tag.
Definition: tags.h:277
bool visible
Whether or not the field is visible.
Definition: fields.h:80
virtual bool ParseXMLData(rapidxml::xml_node<> *field_node)
Populates data within the plugin field from an XML node.
Definition: BlamPluginField.cpp:106
std::string field_id
The ID of the field.
Definition: fields.h:83
BlamPluginField * plugin_field
The plugin data associated with this field, if any.
Definition: fields.h:242
std::string description
An optional description of the field.
Definition: fields.h:82
BlamPlugin * GetPlugin()
Retrieves the plugin this field is associated with.
Definition: BlamPluginField.cpp:36
virtual std::string GenerateCppClassString(std::string line_prefix="")
Generates a string containing C++ code representing this plugin field.
Definition: BlamPluginField.cpp:101
virtual std::string GenerateCppStructString(std::string line_prefix="", bool generate_doxygen_comments=true)
Generates a string containing C++ code representing this plugin field.
Definition: BlamPluginField.cpp:96
virtual std::vector< char > GetValueAsBytes()
Retrieves the field value as a list of bytes.
Definition: BlamTagField.cpp:14