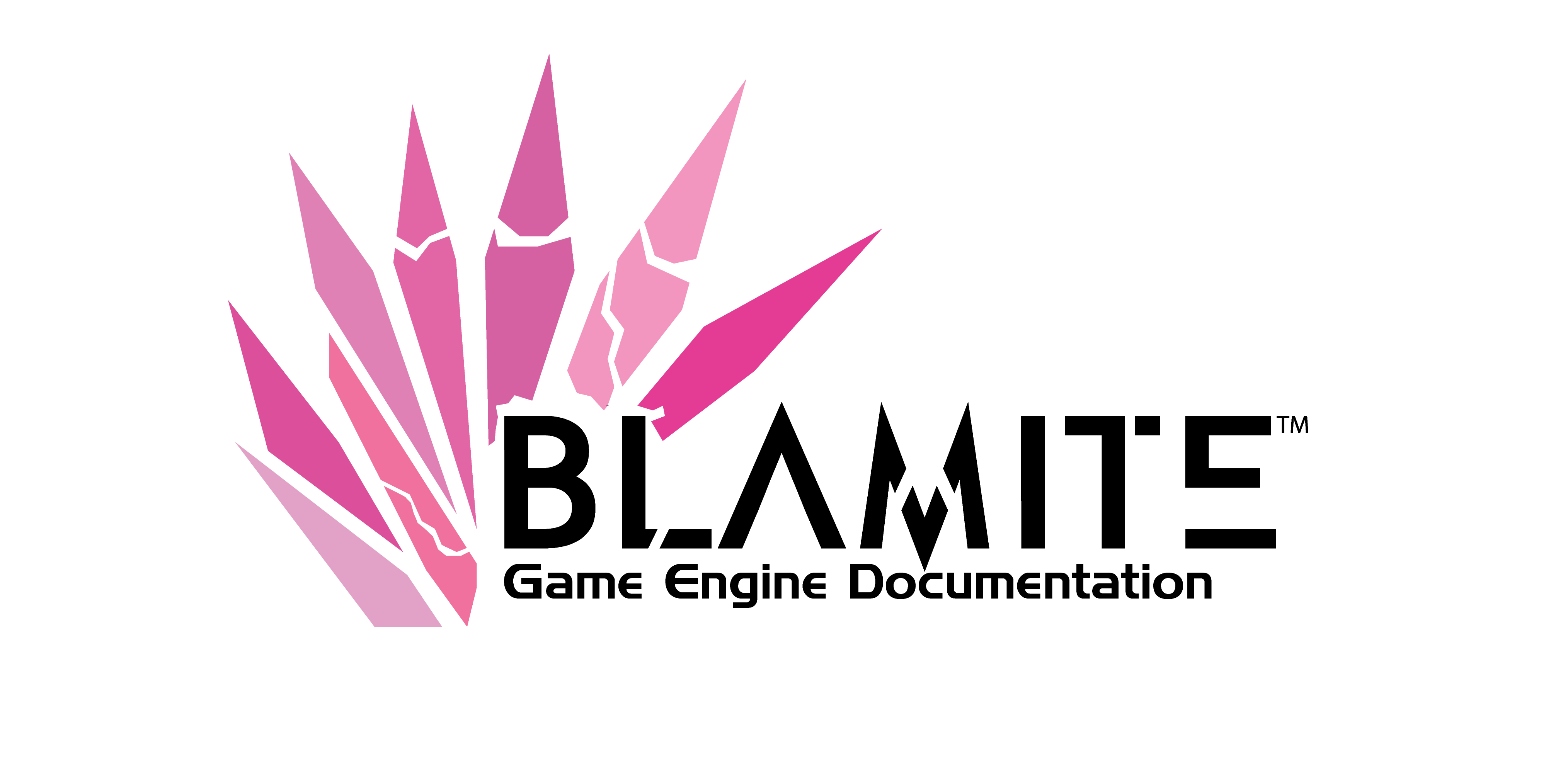 |
Blamite Game Engine - Keystone
00402.09.29.23.0627.blamite
A library that enables the use of Qt in Blamite's editing tools.
|
Go to the documentation of this file.
3 #include <qtreewidget.h>
4 #include <HEKGuerilla/components/projects/projects.h>
17 BlamProject* project =
nullptr;
19 bool is_valid =
false;
20 bool use_hierarchy_view =
true;
22 BlamPlugin* plugin =
nullptr;
23 bool is_fake_container =
false;
30 void GenerateTreeWidgets();
108 void Filter(std::string search_filter,
bool keep_empty_folders);
Interface class that all main editing tool windows inherit from.
Definition: BlamEditorWindow.h:48