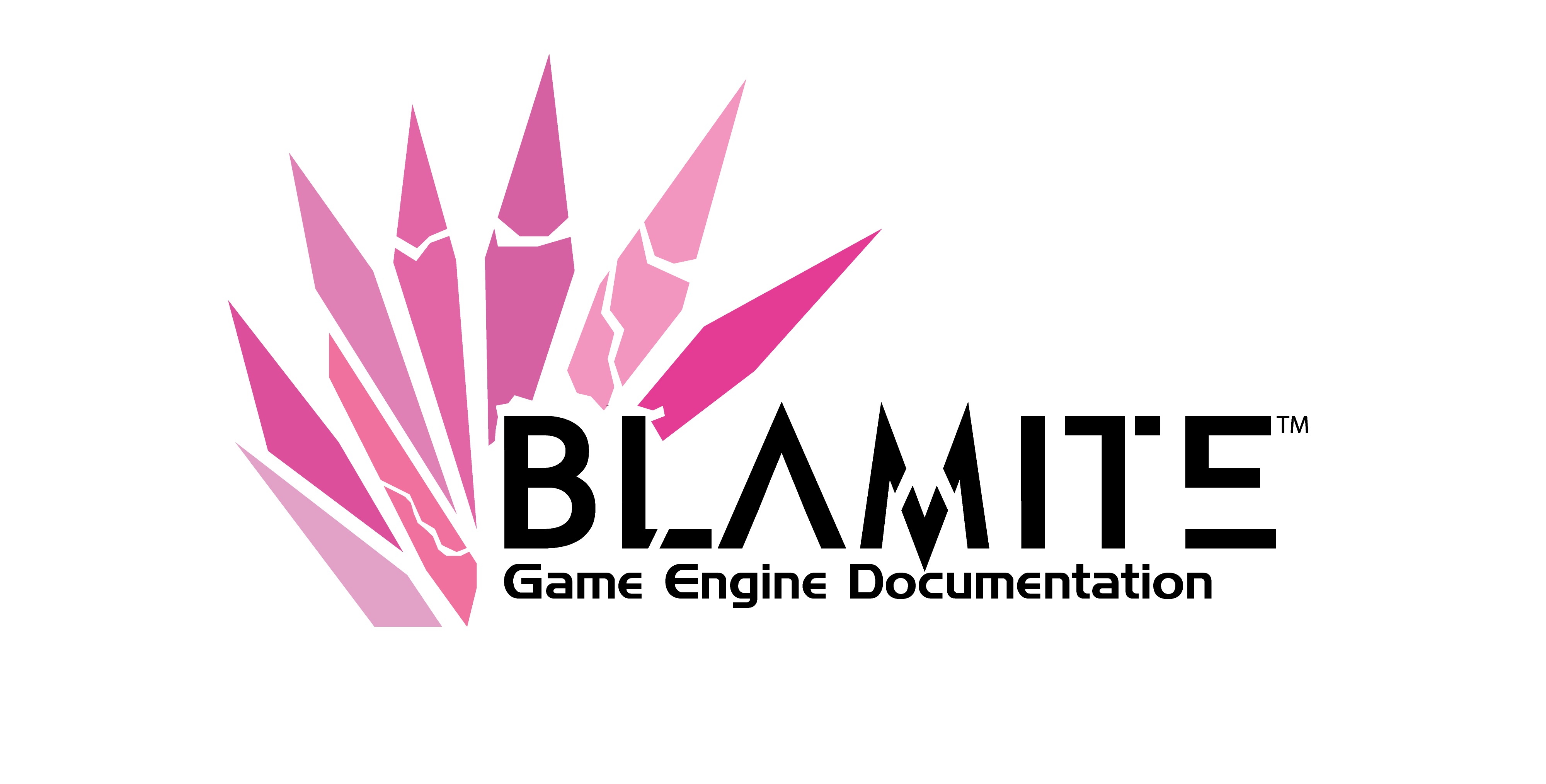 |
Blamite Game Engine - Guerilla (Library)
00421.06.29.24.2305.blamite
The tag editor for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include <Strings/components/resources/project_info/project_info.h>
6 #ifdef GUERILLA_LIB_EXPORTS
7 #define GUERILLA_LIB_API __declspec(dllexport)
9 #define GUERILLA_LIB_API __declspec(dllimport)
15 #define PROJECT_FILE_EXTENSION ".blam"
18 #define BLAM_PROJECTS_DIR "Projects"
47 bool is_folder =
false;
48 bool path_exists =
false;
54 std::string hierarchy_path =
"";
55 std::string disk_path =
"";
56 std::string display_name =
"";
87 std::vector<BlamTagTreeNode*> GetBulkNodeList();
103 bool project_load_result =
false;
105 std::string project_name =
"unnamed";
106 std::string project_root =
"";
119 void BuildTagHierarchy();
121 std::string GetContentRoot();
122 std::string GetTagsRoot();
123 std::string GetMoviesRoot();
124 std::string GetDataRoot();
125 std::string GetCacheRoot();
126 std::string GetPluginsRoot();
127 std::string GetFontsRoot();
std::string GetCacheRoot()
Definition: BlamProject.cpp:200
std::string GetContentRoot()
Definition: BlamProject.cpp:148
std::string disk_path
The path of this node on disk, relative or absolute, ex: ./tags/levels/multi/forge_halo
Definition: projects.h:55
std::vector< BlamTagTreeNode * > children
Any child nodes within this node, will only apply for Folder nodes.
Definition: projects.h:52
GUERILLA_LIB_API BlamProject * LoadProject(std::string file_path)
Loads a project file from disk and prepares it for use.
Definition: projects.cpp:23
std::string GetTagsRoot()
Definition: BlamProject.cpp:161
Class representing a Blamite Project.
Definition: projects.h:97
BlamTagTreeNode(BlamProject *_project, std::string file_path, bool ignore_folder_name_in_hierarchy, BlamTagTreeNode *parent=nullptr)
Constructs a new tag tree node.
Definition: BlamTagTreeNode.cpp:8
GUERILLA_LIB_API std::vector< BlamProject * > GetLoadedProjects()
Retrieves the list of loaded projects.
Definition: projects.cpp:94
BlamProject * active_project
Definition: projects.cpp:11
BlamPlugin * plugin
The plugin/tag class this tag uses. Will be nullptr if the node is a directory or if the tag has no p...
Definition: projects.h:57
BlamProjectInfo project_info
Definition: projects.h:102
std::string GetDataRoot()
Definition: BlamProject.cpp:187
bool path_exists
Whether or not the path associated with this node exists.
Definition: projects.h:48
std::string GetPluginsRoot()
Definition: BlamProject.cpp:213
void Refresh()
Refreshes the node for any changes to its children on disk.
Definition: BlamTagTreeNode.cpp:79
#define GUERILLA_LIB_API
Definition: projects.h:9
Namespace containing functions for working with project files.
Definition: projects.h:133
GUERILLA_LIB_API void SetCurrentProject(BlamProject *project)
Definition: projects.cpp:18
std::string display_name
The display name of this node, ex: forge_halo
Definition: projects.h:56
bool project_load_result
Whether or not the project directory exists and is ready for use.
Definition: projects.h:103
BlamProject(std::string file_path)
Initializes and loads an existing project.
Definition: BlamProject.cpp:8
void BuildTagHierarchy()
Builds the tag hierarchy for the project.
Definition: BlamProject.cpp:126
BlamTagTreeNode * hierarchy_root
The root node for the project's tag hierarchy.
Definition: projects.h:100
std::vector< BlamPlugin * > plugins
The list of loaded plugins.
Definition: plugins.cpp:9
~BlamTagTreeNode()
Destroys a tag tree node.
Definition: BlamTagTreeNode.cpp:74
std::string GetFontsRoot()
Definition: BlamProject.cpp:226
std::string GetMoviesRoot()
Definition: BlamProject.cpp:174
bool is_folder
Indicates whether the node represents a file. If this is false, then the node represents a file.
Definition: projects.h:47
GUERILLA_LIB_API void UnloadProjects()
Releases all project data loaded into memory.
Definition: projects.cpp:84
Class representing a Plugin.
Definition: tags.h:80
BlamProject * project
The project this node belongs to.
Definition: projects.h:50
std::string project_root
The root folder for the project.
Definition: projects.h:106
std::vector< BlamProject * > loaded_projects
Definition: projects.cpp:10
std::string project_name
The name of the project.
Definition: projects.h:105
BlamTagTreeNode * parent
The parent node of this node. If this item is the root node, then this will be nullptr.
Definition: projects.h:51
std::string hierarchy_path
The path of this node within the hierarchy, ex: /levels/multi/forge_halo
Definition: projects.h:54
#define PROJECT_FILE_EXTENSION
Definition: projects.h:15
Class representing a tag tree node.
Definition: projects.h:26
#define BLAM_PROJECTS_DIR
Definition: projects.h:18
GUERILLA_LIB_API void LoadProjects()
Attempts to load all available project folders.
Definition: projects.cpp:40
~BlamProject()
Definition: BlamProject.cpp:121
GUERILLA_LIB_API BlamProject * GetCurrentProject()
Definition: projects.cpp:13
std::vector< BlamTagTreeNode * > GetBulkNodeList()
Retrieves a bulk list of all tag nodes within this node.
Definition: BlamTagTreeNode.cpp:141