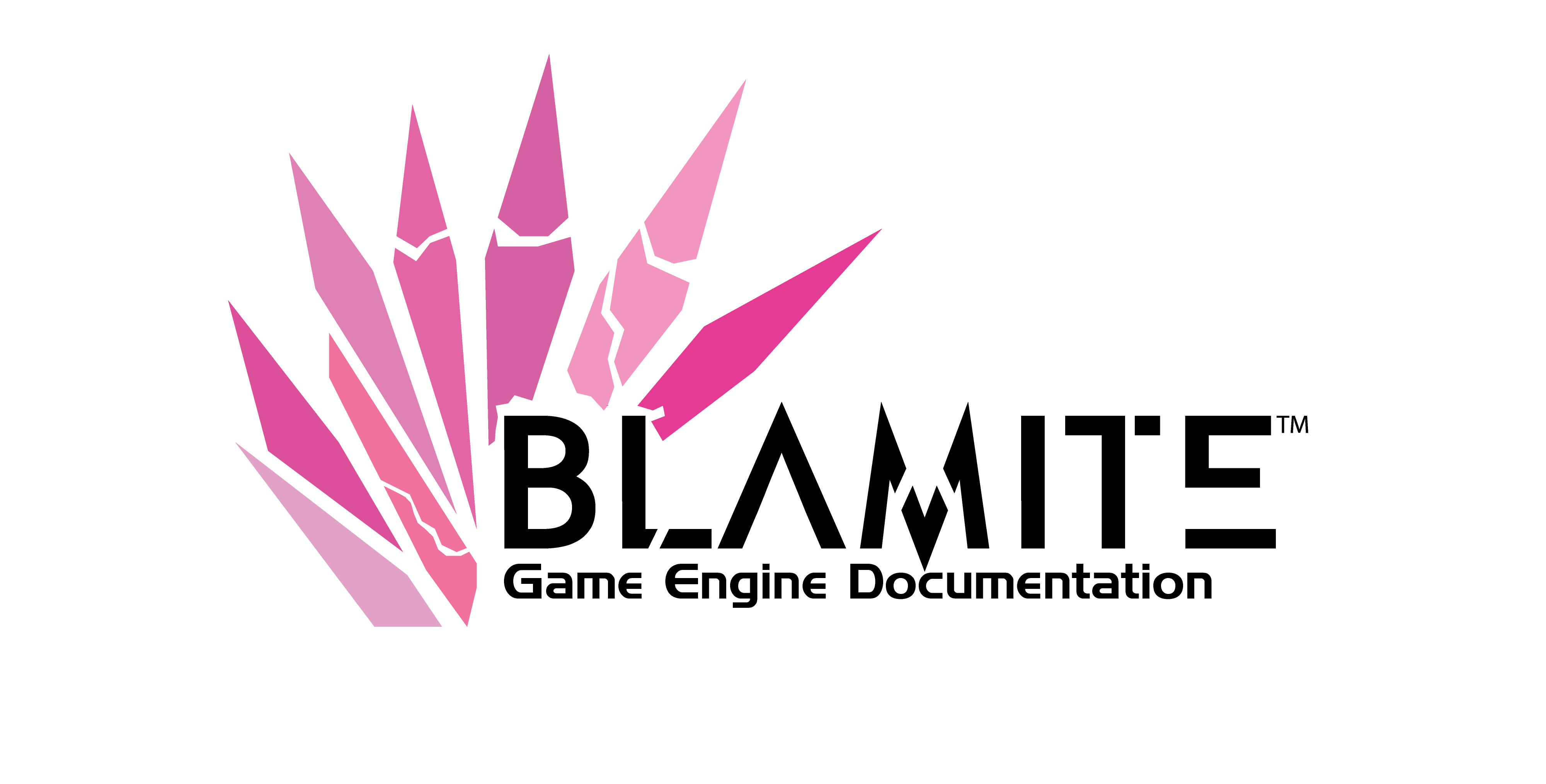 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
7 #include <vulkan/vulkan.h>
8 #include <vulkan/vulkan_win32.h>
10 #include <glew/glew.h>
16 #include "components/3rdparty/imgui/imgui.h"
45 namespace DebugMessenger
138 namespace Presentation
656 BLAM void ShowCrashScreen(
const char* exc,
const char* file,
int line, std::string message);
Namespace surrounding all major engine components.
Definition: blam_api.h:18
BLAM void SetClearColor(ImVec4 color)
Changes the color to used to clear the render target.
Definition: rendering_abstraction.cpp:26
BLAM HRESULT ConvertWICBitmapToD2D(IWICBitmap *wic_bitmap, ID2D1Bitmap **d2d_bitmap)
Converts a WIC bitmap to a D2D bitmap.
Definition: wic.cpp:91
BLAM VkQueue * GetPresentQueue()
Retrieves the Vulkan presentation queue.
Definition: presentation.cpp:45
BLAM ImVec4 * GetClearColor()
Retrieves the current color used to clear the render target.
Definition: render_manage.cpp:613
BLAM VkInstance * GetInstance()
Retrieves the Vulkan instance.
Definition: vulkan.cpp:28
BLAM void SelectManualDevice(int device_index)
Instructs Vulkan to try to select a specific device.
Definition: device.cpp:145
BLAM void SetViewClearFlags(int new_flags)
Definition: bgfx.cpp:137
BLAM void ShutdownRenderThread()
Instructs the rendering thread to stop running.
Definition: render_manage.cpp:331
BLAM HRESULT GetLastFrameTextureAlt(ID3D11Texture2D *texture)
Retrieves the last frame that was rendered.
Definition: render_manage.cpp:647
int width
Definition: bgfx.cpp:18
BLAM HRESULT ConvertWICBitmapTo32bppPBGRA(IWICBitmap *source, IWICBitmap **destination)
Converts a WIC bitmap to a WIC bitmap, with the 32 bits-per-pixe, PBGRA format.
Definition: wic.cpp:58
LPSTR details
Definition: error_notice.cpp:17
BLAM BlamRenderingEngine GetCurrentRenderingEngine()
Retrieves the current rendering engine being used.
Definition: rendering_abstraction.cpp:103
BLAM void Shutdown()
Shuts down the Vulkan debug messenger.
Definition: debug_messenger.cpp:158
BLAM void HandleWindowResize(int width, int height, WPARAM wParam)
Definition: rendering_abstraction.cpp:80
BLAM void CreateSurface()
Prepares the Vulkan surface used for rendering.
Definition: presentation.cpp:9
BLAM IDWriteFactory * GetDWriteFactory()
Retrieves the DirectWrite factory.
Definition: render_manage.cpp:573
BlamRenderingEngine
Definition: rendering.h:23
BLAM void Shutdown()
Shuts down OpenGL.
Definition: opengl.cpp:157
BLAM ID2D1DeviceContext * GetD2DRenderTarget()
Retrieves the Direct2D render target.
Definition: render_manage.cpp:598
BLAM void ShowCrashScreen(const char *exc, const char *file, int line, std::string message)
Displays the engine crash screen.
Definition: rendering_abstraction.cpp:61
BLAM HRESULT SetDisplayRes(int x, int y)
Changes the game's display resolution.
Definition: rendering_abstraction.cpp:7
BLAM void DisplaySignalCrashScreen(int signal)
Displays a signal-based engine crash screen.
Definition: render_manage.cpp:464
BLAM void SetClearColor(ImVec4 color)
Changes the color to used to clear the render target.
Definition: render_manage.cpp:567
BLAM void CreateLogicalDevice()
Definition: device.cpp:252
BLAM void DisplayDetailedCrashScreen(const char *expression, const char *file, int line, std::string details)
Displays a detailed engine crash screen.
Definition: render_manage.cpp:443
BLAM std::vector< VkExtensionProperties > GetAvailableExtensions()
Retrieves the list of all extensions available to Vulkan.
Definition: vulkan.cpp:225
BLAM HRESULT CreateWICBitmapFromMemory(void *data, int size, IWICBitmap **bitmap_pointer)
Creates a WIC bitmap from a block of memory.
Definition: wic.cpp:173
@ color
Definition: render_model.h:12
BLAM void HandleWindowReisze(int width, int height)
Definition: bgfx.cpp:127
BLAM HRESULT GetLastFrameHResult()
Unused.
Definition: render_manage.cpp:642
BLAM HRESULT Initialize(HWND hWnd)
Initializes DirectX 11.
Definition: render_manage.cpp:74
BLAM HRESULT UpdateResolution(int x, int y)
Changes the DirectX render target resolution.
Definition: resolution.cpp:9
BLAM ImVec4 * GetClearColor()
Retrieves the color used for render target clearing.
Definition: rendering_abstraction.cpp:45
BLAM void Shutdown()
Definition: bgfx.cpp:118
BLAM void RenderLoop()
Renders engine graphics onscreen.
Definition: vulkan.cpp:295
BLAM HRESULT Initialize()
Initializes the Windows Imaging Component (WIC).
Definition: wic.cpp:12
BLAM ID3D11Texture2D ** GetLastFrameTexture()
Retrieves the last frame that was rendered.
Definition: render_manage.cpp:626
BLAM bool ValidationLayersEnabled()
Checks whether or not validation layers are enabled.
Definition: vulkan.cpp:33
BLAM bool CheckValidationLayerSupport()
Checks whether or not Vulkan's default validation layer is available.
Definition: vulkan.cpp:195
BLAM HRESULT LoadFontFromFile(std::string id, std::string file_path)
Loads a TrueType font from the specified file into the list of loaded font collections.
Definition: render_manage.cpp:555
BLAM DXGI_SWAP_CHAIN_DESC GetSwapChainDesc()
Retrieves the current Direct3D Swap Chain description.
Definition: render_manage.cpp:618
BLAM void Initialize()
Initializes Vulkan.
Definition: vulkan.cpp:247
BLAM void SelectDefaultDevice()
Instructs Vulkan to select the first compatiable device.
Definition: device.cpp:213
BLAM HRESULT HandleWindowResize(LPARAM lParam)
Handles a window resize event.
Definition: render_manage.cpp:306
BLAM void TakeScreenshot()
Captures the current frame and saves it to a file.
Definition: screenshot.cpp:100
BLAM ID2D1Factory * GetD2DFactory()
Retrieves the Direct2D factory.
Definition: render_manage.cpp:603
BLAM int RateDeviceSuitability(VkPhysicalDevice device)
Rates a given physical device based on its capabilities and features.
Definition: device.cpp:117
BLAM void RenderLoop()
Definition: bgfx.cpp:66
BLAM void SetPlatformData()
BLAM std::vector< VkPhysicalDevice > GetDeviceList()
Retrieves the list of physical devices available to Vulkan.
Definition: device.cpp:92
BLAM void ScreenshotDone()
Informs DirectX that the screenshot has finished being captured.
Definition: render_manage.cpp:664
BLAM void PopulateCreateInfo(VkDebugUtilsMessengerCreateInfoEXT *create_info)
Populates debug messenger creation information.
Definition: debug_messenger.cpp:79
BLAM std::vector< const char * > GetRequiredExtensions()
Gets a list of required Vulkan extensions.
Definition: vulkan.cpp:178
BLAM void Initialize()
Initializes the Vulkan debug messenger.
Definition: debug_messenger.cpp:119
@ Vulkan
Definition: rendering.h:26
BLAM HRESULT CreateD2DBitmapFromResource(int res_id, const char *res_type, ID2D1Bitmap **bitmap_pointer)
Creates a Direct2D bitmap from an application resource.
Definition: wic.cpp:262
BLAM ID3D11RenderTargetView * GetD3DRenderTargetView()
Retrieves the current Direct3D Render Target.
Definition: render_manage.cpp:593
BLAM IDXGISwapChain * GetDXGISwapChain()
Retrieves the current DXGI Swap Chain.
Definition: render_manage.cpp:588
BLAM void DisplayBasicCrashScreen()
Displays a basic engine crash screen.
Definition: render_manage.cpp:456
BLAM ID3D11Device * GetD3DDevice()
Retrieves the current Direct3D device.
Definition: render_manage.cpp:578
@ NotReady
Definition: rendering.h:29
BLAM HRESULT CreateWICBitmapFromFile(std::wstring path, IWICBitmap **bitmap_pointer)
Creates a WIC bitmap from a file.
Definition: wic.cpp:141
@ DirectX11
Definition: rendering.h:25
BLAM void DestroySurface()
Destroys the Vulkan surface.
Definition: presentation.cpp:33
int height
Definition: bgfx.cpp:19
Namespace for things relating to rendering.
Definition: models.h:150
BLAM void RenderLoop()
Renders a frame to the application window.
Definition: opengl.cpp:132
BLAM std::vector< VkLayerProperties > GetAvailableLayers()
Retrieves the list of all validation layers available to Vulkan.
Definition: vulkan.cpp:236
BLAM void DestroyLogicalDevice()
Definition: device.cpp:308
BLAM BlamResult Initialize()
Initializes OpenGL.
Definition: opengl.cpp:69
BLAM void RenderLoop(bool debug)
Renders everything to the screen.
Definition: render_manage.cpp:370
BLAM ID3D11DeviceContext * GetD3DContext()
Retrieves the current Direct3D context.
Definition: render_manage.cpp:583
BLAM IWICImagingFactory * GetWICFactory()
Retrieves the WIC Imaging Factory.
Definition: wic.cpp:53
BLAM void PrintVulkanAvailabilityInfo()
Prints lists of available Vulkan extensions and validation layers.
Definition: vulkan.cpp:43
BLAM bool IsDeviceSuitable(VkPhysicalDevice device)
Determines whether or not a given Vulkan device is suitable for the engine's requirements.
Definition: device.cpp:103
BLAM void SelectBestDevice()
Instructs Vulkan to select the best compatiable device.
Definition: device.cpp:169
BLAM void Shutdown()
Shuts down Vulkan.
Definition: vulkan.cpp:300
#define BLAM
Definition: rendering.h:20
BLAM std::vector< const char * > GetEnabledLayers()
Retrieves the list of all enabled Vulkan layers.
Definition: vulkan.cpp:38
BLAM void Shutdown()
Shuts down the Windows Imaging Component (WIC).
Definition: wic.cpp:47
BLAM void Cleanup()
Cleans up all DirectX data.
Definition: render_manage.cpp:237
BLAM void Initialize()
Definition: bgfx.cpp:26
BLAM bool HasRenderThreadStopped()
Determines whether or not the render thread has finished stopping.
Definition: render_manage.cpp:326
BLAM bool * RenderTargetClearing()
Retrieves whether or not to enable render target clearing.
Definition: render_manage.cpp:608
@ BGFX
Definition: rendering.h:28
BLAM SDL_GLContext * GetGLContext()
Retrieves the current OpenGL context.
Definition: opengl.cpp:173
BLAM VkSurfaceKHR * GetSurface()
Retrieves the Vulkan window surface.
Definition: presentation.cpp:28
@ OpenGL3
Definition: rendering.h:27
BLAM HRESULT CreateD2DBitmapFromFile(std::wstring path, ID2D1Bitmap **bitmap_pointer)
Creates a Direct2D bitmap from a file.
Definition: wic.cpp:216
BLAM void RenderThread(bool debug)
Instructs the engine to start rendering on a separate thread.
Definition: render_manage.cpp:337