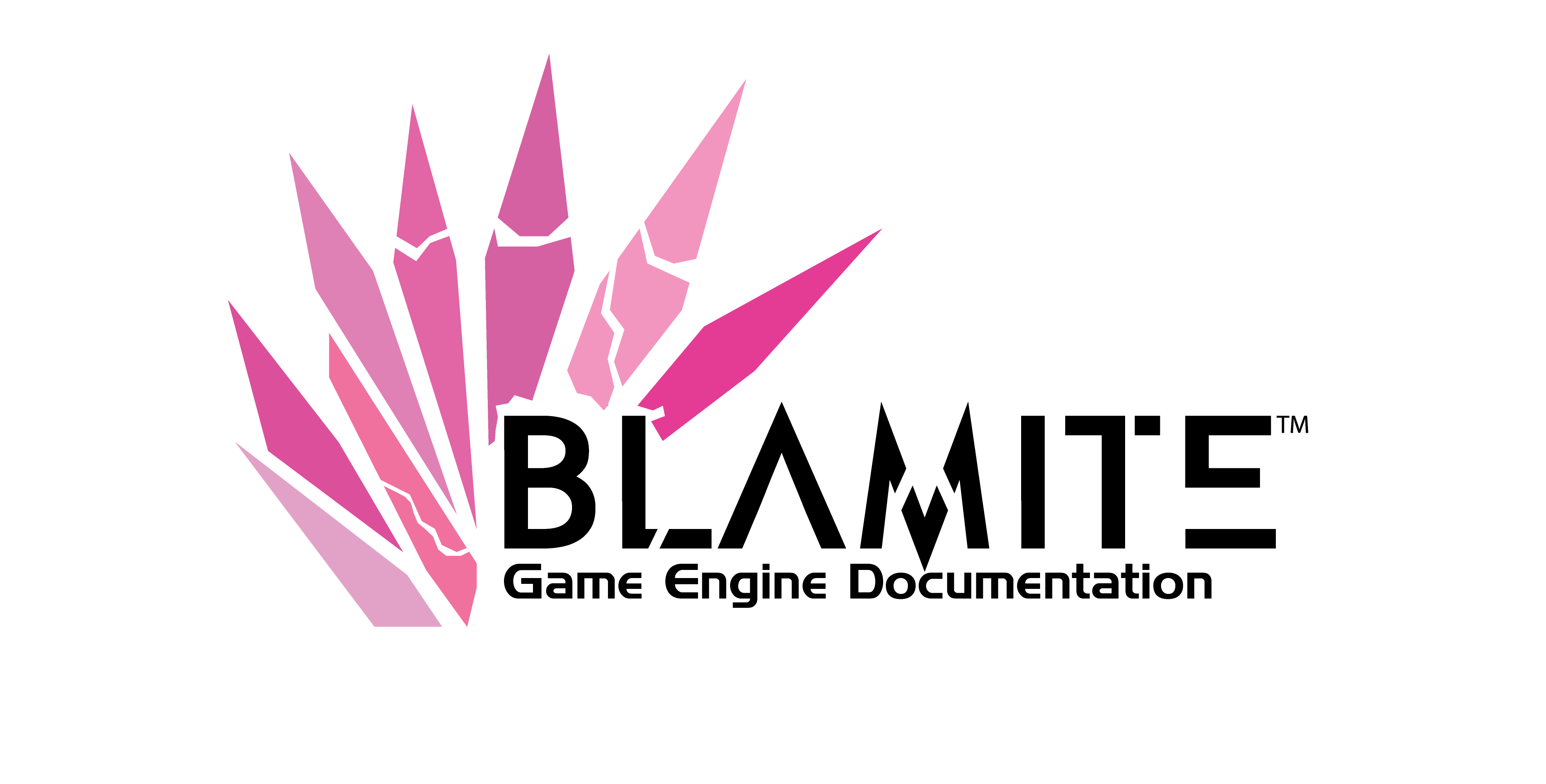 |
Blamite Game Engine - blam!
00346.12.11.21.0529.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../../debug_ui.h"
15 int active_widget_index = -1;
17 bool show_add_widget_dialog =
false;
18 bool add_widget_to_group =
false;
20 std::string widget_type_name =
"rectangle";
45 widget_type_name =
"basic_rectangle";
57 widget_type_name =
"group";
92 show_add_widget_dialog =
false;
99 show_add_widget_dialog =
false;
115 std::string window_title =
"";
123 window_title =
"Blam UI Editor (no root available, root was nullptr)";
132 show_add_widget_dialog =
true;
135 if (active_widget_index >= 0 && active_widget_index < ui_root->children.size())
143 delete widget_to_remove;
145 active_widget_index = -1;
148 if ((active_widget_index - 1) >= 0)
154 int new_pos = active_widget_index - 1;
161 active_widget_index--;
171 int new_pos = active_widget_index + 1;
178 active_widget_index++;
194 std::string selectable_item_name = std::to_string(i) +
": " + widget->
display_name;
195 bool is_active_item =
false;
197 if (i == active_widget_index)
199 is_active_item =
true;
204 active_widget_index = i;
214 std::string display_name =
"display name: " + widget->
display_name;
215 std::string description =
"description: " + widget->
description;
216 std::string index =
"index: " + std::to_string(i);
232 if (active_widget_index < 0)
238 std::string message =
"selected index " + std::to_string(active_widget_index) +
239 " was too high (max is " + std::to_string(
ui_root->
children.size()) +
")";
251 std::string display_name =
"display name: " + widget->
display_name;
252 std::string description =
"description: " + widget->
description;
253 std::string index =
"index: " + std::to_string(active_widget_index);
277 if (show_add_widget_dialog)
IMGUI_API void BeginTooltip()
Definition: imgui.cpp:7362
IMGUI_API void EndPopup()
Definition: imgui.cpp:7675
void ShowAddPrimitivePopup()
Definition: blam_ui_editor.hpp:35
BLAM BlamUIWidget_Group * GetUIRoot()
Definition: ui.cpp:47
IMGUI_API bool BeginPopupModal(const char *name, bool *p_open=NULL, ImGuiWindowFlags flags=0)
Definition: imgui.cpp:7647
IMGUI_API void ListBoxFooter()
Definition: imgui_widgets.cpp:5668
IMGUI_API void NextColumn()
Definition: imgui_widgets.cpp:7445
BLAM float * GetGlobalAsFloat(std::string name)
Retrieves a global's value as a float.
Definition: globals.cpp:407
IMGUI_API void End()
Definition: imgui.cpp:6016
IMGUI_API void SetColumnWidth(int column_index, float width)
Definition: imgui_widgets.cpp:7297
IMGUI_API void EndTabItem()
Definition: imgui_widgets.cpp:6851
IMGUI_API void SameLine(float offset_from_start_x=0.0f, float spacing=-1.0f)
Definition: imgui.cpp:7147
BlamUIEditor()
Definition: blam_ui_editor.hpp:25
BlamUIEditor(BlamUIWidget_Group *_ui_root)
Definition: blam_ui_editor.hpp:30
void Draw()
Draws the contents of the group.
Definition: blam_ui_editor.hpp:106
IMGUI_API bool IsItemHovered(ImGuiHoveredFlags flags=0)
Definition: imgui.cpp:3061
IMGUI_API bool Begin(const char *name, bool *p_open=NULL, ImGuiWindowFlags flags=0)
Definition: imgui.cpp:5397
IMGUI_API void OpenPopup(const char *str_id)
Definition: imgui.cpp:7453
IMGUI_API float GetColumnWidth(int column_index=-1)
Definition: imgui_widgets.cpp:7262
IMGUI_API bool BeginTabBar(const char *str_id, ImGuiTabBarFlags flags=0)
Definition: imgui_widgets.cpp:6366
IMGUI_API void Text(const char *fmt,...) IM_FMTARGS(1)
Definition: imgui_widgets.cpp:238
IMGUI_API void PopTextWrapPos()
Definition: imgui.cpp:6313
IMGUI_API void EndTooltip()
Definition: imgui.cpp:7402
IMGUI_API bool BeginCombo(const char *label, const char *preview_value, ImGuiComboFlags flags=0)
Definition: imgui_widgets.cpp:1416
IMGUI_API bool Selectable(const char *label, bool selected=false, ImGuiSelectableFlags flags=0, const ImVec2 &size=ImVec2(0, 0))
Definition: imgui_widgets.cpp:5469
bool show
Controls whether or not the group should be shown. May not be used in all groups.
Definition: debug_ui.h:362
Class representing an ImGUI drawing group/draw list item.
Definition: debug_ui.h:359
IMGUI_API void Columns(int count=1, const char *id=NULL, bool border=true)
Definition: imgui_widgets.cpp:7572
Class for the Blam UI Editor debug utility.
Definition: blam_ui_editor.hpp:12
IMGUI_API void EndCombo()
Definition: imgui_widgets.cpp:1522
BlamUIWidget_Group * ui_root
Definition: ui.cpp:6
IMGUI_API void EndTabBar()
Definition: imgui_widgets.cpp:6432
IMGUI_API void Separator()
Definition: imgui_widgets.cpp:1284
BlamWidgetType
Definition: ui.h:40
Legacy namespace to contain data for the legacy ImGUI console.
Definition: ui.h:14
IMGUI_API void PushTextWrapPos(float wrap_local_pos_x=0.0f)
Definition: imgui.cpp:6306
IMGUI_API bool BeginTabItem(const char *label, bool *p_open=NULL, ImGuiTabItemFlags flags=0)
Definition: imgui_widgets.cpp:6829
IMGUI_API bool ListBoxHeader(const char *label, const ImVec2 &size=ImVec2(0, 0))
Definition: imgui_widgets.cpp:5614
IMGUI_API bool Button(const char *label, const ImVec2 &size=ImVec2(0, 0))
Definition: imgui_widgets.cpp:644