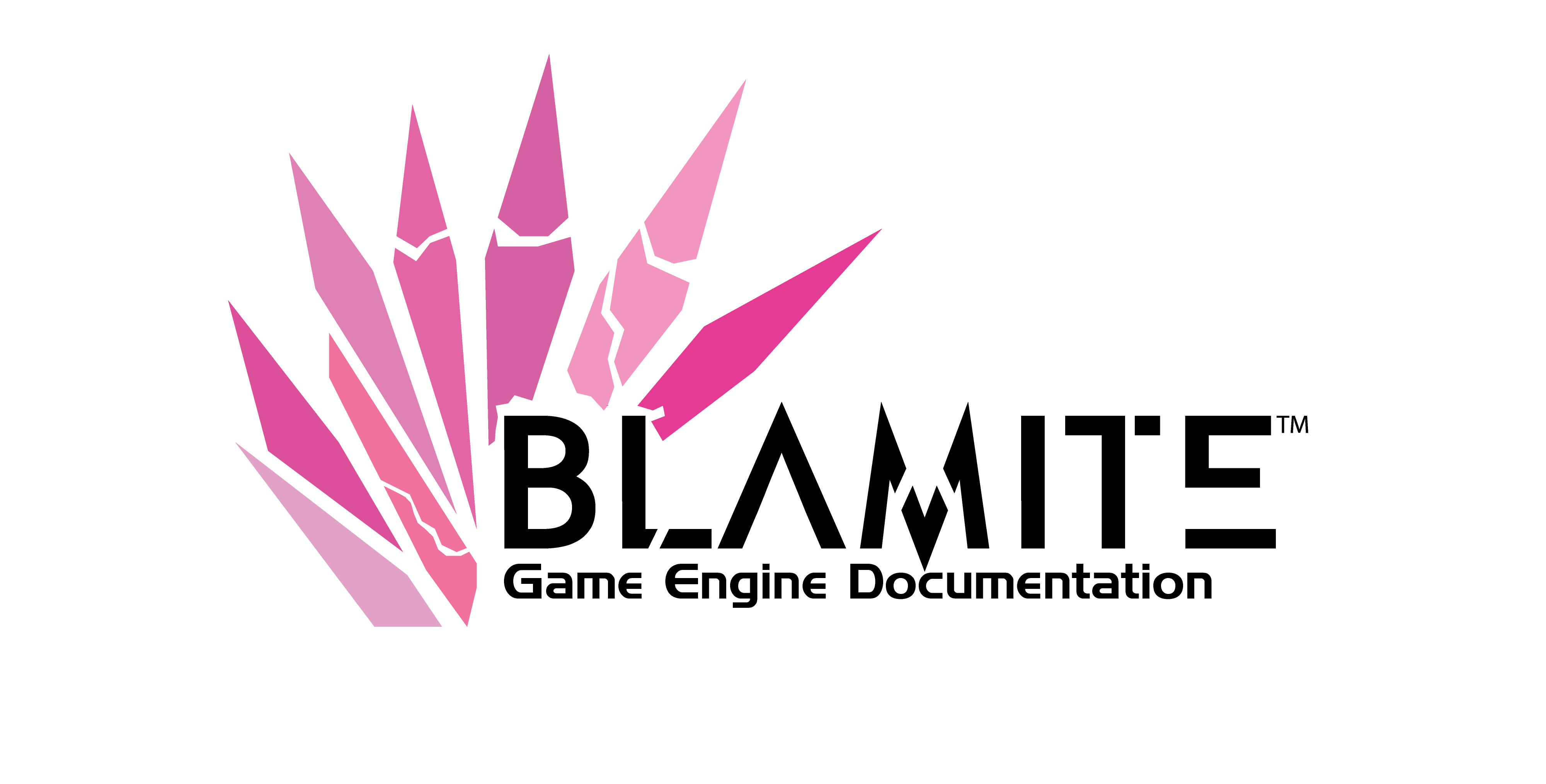 |
Blamite Game Engine - blam!
00296.01.12.21.0102.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../debug_ui.h"
5 #include <Strings/components/utils/io/io.h>
6 #include <Strings/components/utils/utilities.h>
31 bool old_devtools_bar =
false;
32 bool show_menubar =
true;
34 int commit_hash_label = 0;
36 bool show_imgui_font_picker =
false;
37 bool show_imgui_metrics =
false;
38 bool show_imgui_style_picker =
false;
39 bool show_imgui_style_editor =
false;
41 bool main_menu =
true;
93 ImGui::BeginMainMenuBar();
97 ImGui::Begin(
"Final Hardcoded Menu", &
show, ImGuiWindowFlags_MenuBar | ImGuiWindowFlags_AlwaysAutoResize | ImGuiWindowFlags_NoResize);
98 ImGui::BeginMenuBar();
103 if (ImGui::BeginMenu(
"File"))
105 ImGui::MenuItem(
"Open .map file", NULL,
false,
false);
ShowNYITooltip();
106 ImGui::MenuItem(
"Return to mainmenu", NULL,
false,
false);
ShowNYITooltip();
107 if (ImGui::MenuItem(
"Open game dir", NULL)) BlamStrings::Utils::IO::OpenGameDirectory();
109 if (ImGui::MenuItem(
"Open Report Viewer", NULL))
114 BlamStrings::Utils::OpenLocalWebURL(report_viewer_page);
117 ImGui::MenuItem(
"Exit", NULL,
duigvs(
"exit_via_menu"));
120 if (ImGui::BeginMenu(
"Tools"))
124 if (ImGui::BeginMenu(
"Network"))
126 ImGui::MenuItem(
"Show Network Info", NULL,
duigvs(
"network_stats"));
127 ImGui::MenuItem(
"Discord Rich Presence", NULL,
duigvs(
"discord_rpc_ui"));
131 if (ImGui::BeginMenu(
"Rendering"))
133 ImGui::MenuItem(
"Render Stack Editor", NULL,
duigvs(
"render_stack_editor"));
134 ImGui::MenuItem(
"Font Editor", NULL,
duigvs(
"font_editor"));
139 if (ImGui::BeginMenu(
"Test"))
141 if (ImGui::BeginMenu(
"Deprecated"))
143 ImGui::TextColored(ImVec4(1, 0, 0, 1),
ENGINE_TEXT(
"imgui_menu_deprecated_warning").c_str());
145 ImGui::MenuItem(
"Crash Test", NULL,
duigvs(
"crash_test_ui"));
146 ImGui::MenuItem(
"Access Key Dialog", NULL,
duigvs(
"license_startup_prompt"));
147 ImGui::MenuItem(
"Old Menu", NULL, &old_devtools_bar);
152 if (ImGui::BeginMenu(
"Rendering"))
154 ImGui::MenuItem(
"Direct2D Test Window", NULL,
duigvs(
"d2d_test"));
155 ImGui::MenuItem(
"bgfx Test Window", NULL,
duigvs(
"bgfx_test"));
156 ImGui::MenuItem(
"Geometry Test", NULL,
duigvs(
"geometry_test"));
162 ImGui::MenuItem(
"Default Example Window", NULL,
duigvs(
"dx11_window"));
168 if (ImGui::BeginMenu(
"ImGUI"))
170 ImGui::MenuItem(
"Font Selector", NULL, &show_imgui_font_picker);
171 ImGui::MenuItem(
"Metrics", NULL, &show_imgui_metrics);
172 ImGui::MenuItem(
"Style Selector", NULL, &show_imgui_style_picker);
173 ImGui::MenuItem(
"Style Editor", NULL, &show_imgui_style_editor);
183 ImGui::MenuItem(
"Engine Text Viewer", NULL,
duigvs(
"engine_text_viewer"));
187 ImGui::MenuItem(
"Tag Editor", NULL,
duigvs(
"tag_editor"));
188 ImGui::MenuItem(
"Globals Editor", NULL,
duigvs(
"globals_editor"));
192 ImGui::MenuItem(
"Director Debug", NULL,
duigvs(
"director_tool"));
193 ImGui::MenuItem(
"Execute Command", NULL,
duigvs(
"imgui_console"));
197 if (ImGui::BeginMenu(
"Network Tools"))
202 if (ImGui::BeginMenu(
"Debug"))
204 if (ImGui::MenuItem(
"Debug Menu",
"PgUp"))
208 if (debug_menu_stack_item)
217 ImGui::MenuItem(
"Debug Menu (imgui)",
"Shift + PgUp",
duigvs(
"debug_menu"));
220 if (ImGui::BeginMenu(
"Settings"))
222 ImGui::MenuItem(
"Configuration Editor", NULL,
false,
false);
ShowNYITooltip();
223 ImGui::MenuItem(
"Theme Selector", NULL,
duigvs(
"theme_selector"));
224 ImGui::MenuItem(
"Theme Editor", NULL,
duigvs(
"theme_editor"));
227 if (ImGui::BeginMenu(
"Monitoring"))
229 ImGui::MenuItem(
"Show FPS", NULL,
false,
false);
ShowNYITooltip();
231 ImGui::MenuItem(
"Show Usage Info", NULL,
false,
false);
ShowNYITooltip();
234 if (ImGui::BeginMenu(
"Help"))
236 ImGui::MenuItem(
"ImGui User Guide", NULL,
duigvs(
"imgui_user_guide"));
238 ImGui::MenuItem(
"About ImGui", NULL,
duigvs(
"imgui_info"));
239 ImGui::MenuItem(
"About Blamite", NULL,
duigvs(
"engine_info_new"));
245 ImGui::SameLine(ImGui::GetWindowWidth() - (commit_hash_label + 15));
249 if (ImGui::IsItemHovered())
251 ImGui::BeginTooltip();
252 ImGui::PushTextWrapPos(450.0f);
253 ImGui::TextUnformatted(
ENGINE_TEXT(
"imgui_menu_commit_tooltip_title").c_str());
257 ImGui::TextUnformatted(
ENGINE_TEXT(
"imgui_menu_commit_tooltip_text").c_str());
260 ImGui::PopTextWrapPos();
264 ImVec2 commit_label_size = ImGui::GetItemRectSize();
266 commit_hash_label = commit_label_size.x;
270 ImGui::EndMainMenuBar();
276 ImGui::Text(
"this is the final iteration of the hardcoded menu bar");
277 ImGui::Text(
"all newer menu bar changes are controlled by menu_bar_init.xml");
284 if (old_devtools_bar)
286 ImGui::Begin(
"Old Menu", &old_devtools_bar, ImGuiWindowFlags_MenuBar | ImGuiWindowFlags_AlwaysAutoResize | ImGuiWindowFlags_NoResize);
290 ImGui::Text(
"can't imagine why you'd need it, but here it is");
295 if (show_imgui_font_picker)
297 ImGui::ShowFontSelector(
"Choose Font");
300 if (show_imgui_metrics)
302 ImGui::ShowMetricsWindow(&show_imgui_metrics);
305 if (show_imgui_style_picker)
307 ImGui::ShowStyleSelector(
"Choose Style");
310 if (show_imgui_style_editor)
312 if (ImGui::Begin(
"ImGui Style Editor", &show_imgui_style_editor))
314 ImGui::ShowStyleEditor();
Class for the default ImGUI DX11 sample window.
Definition: dx11_window.hpp:13
BLAM ConfigFile * GetConfig()
Definition: compat.cpp:5
#define WSV_ERROR
Macro for 'Error' log seveirty. Original pre-enum value was 2.
Definition: logger.h:17
BLAM void LogEventForce(std::string message, BlamLogLevel severity)
Forcibly logs a message to the log and/or console.
Definition: aliases.cpp:218
BLAM void TakeScreenshot()
Captures the current frame and saves it to a file.
Definition: screenshot.cpp:100
bool show
Controls whether or not the group should be shown. May not be used in all groups.
Definition: debug_ui.h:362
Class representing an ImGUI drawing group/draw list item.
Definition: debug_ui.h:359
#define CURRENT_COMMIT
Definition: version_data.h:14
#define ENGINE_TEXT(string_id)
Definition: engine_text.h:7
BLAM ImGUIDrawingGroup * GetDrawListItem(std::string key)
Retrieves a drawing group with the specified ID.
Definition: drawing_list.cpp:30
std::string GetString(std::string option)
Definition: compat.cpp:58
Legacy namespace to contain data for the legacy ImGUI console.
Definition: debug_ui.h:434
@ Text
Master text object that wraps around both BitmapText and DWText.
Definition: render_stack.h:73
BLAM bool * RenderTargetClearing()
Retrieves whether or not to enable render target clearing.
Definition: render_manage.cpp:608
#define duigvs(x)
Macro for Blam::DebugUI::GetVisibility().
Definition: debug_ui.h:19
BLAM StackObjectBase * GetStackItem(std::string id)
Retrieves an item from the render stack.
Definition: render_stack.cpp:75