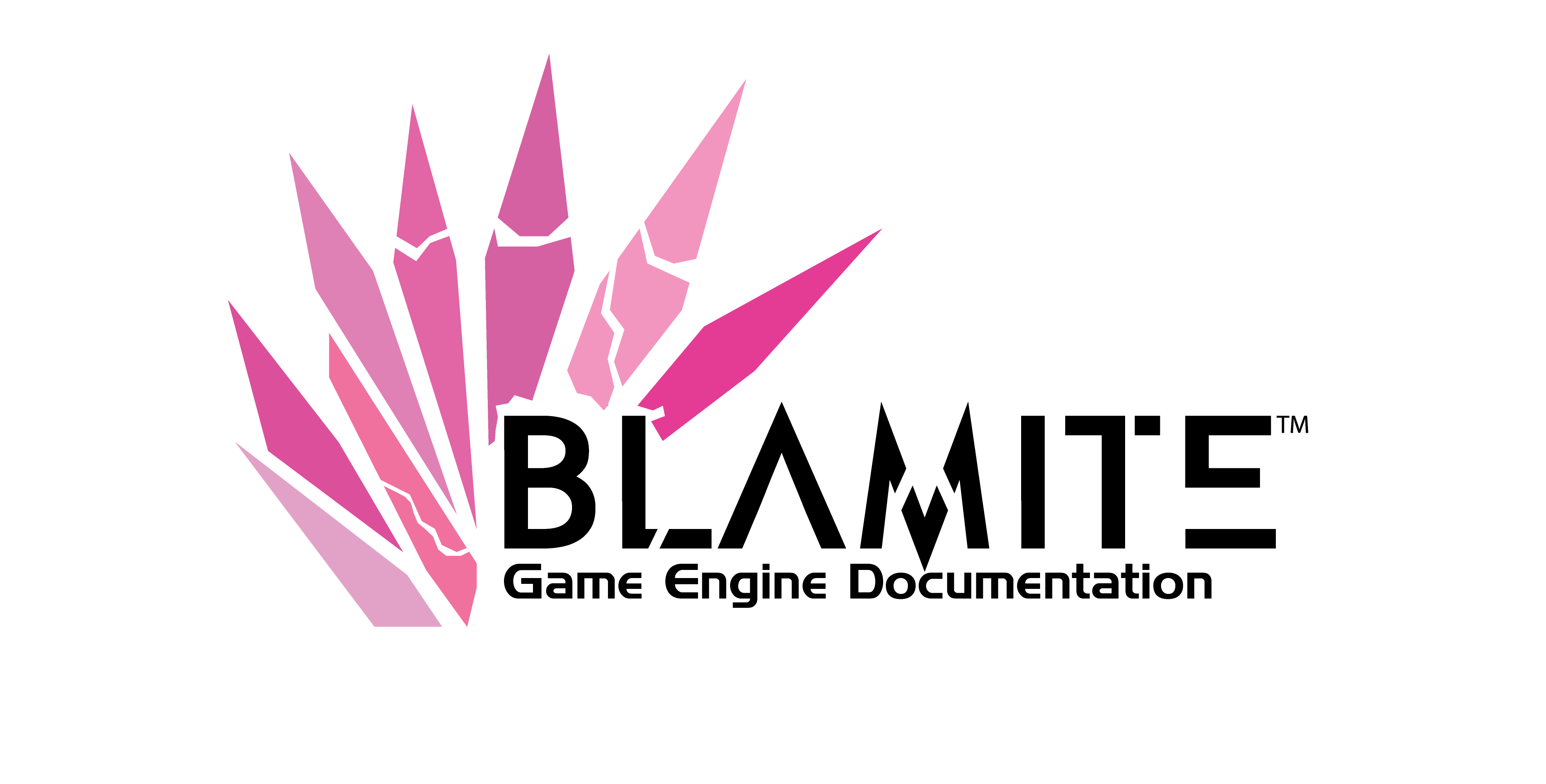 |
Blamite Game Engine - blam!
00346.12.11.21.0529.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
3 #include "../tagclass.h"
89 {1,
"haloman30",
"Initial implementation."},
90 {2,
"haloman30",
"Add test datarefs and tagrefs"}
97 new CommentField(
"Bitmap",
"im gonna shove a bitmap up your ass"),
99 new Int16Field(
"short test",
"A short has a length of 2 bytes."),
100 new Int8Field(
"byte test",
"A byte has a length of 1 bytes."),
101 new BlockField(
sizeof(bitmap::bitmap_data_entry),
"block test",
"*sweats nervously*",
106 new CommentField(
"comment title",
"explanation goes here brotheR"),
114 new Enum32Field(
"type",
"the usage type of this bitmap",
122 new BlockField(
sizeof(bitmap::the_go_fuck_yourself_block_entry),
"the 'go fuck yourself' block",
"yea im tired leave me alone",
126 "can you imagine needing this many flags",
129 new Bitfield32Field(
"who needs this many fucking flags",
"apparently me because i put this shit here",
131 "enable diffusion dithering",
132 "disable height map compression",
133 "uniform sprite sequences",
134 "filthy sprite bug fix",
135 "use sharp bump filter",
137 "use clamped/mirrored bump",
138 "invert detail fade",
139 "swap x-y vector components",
140 "convert from signed",
142 "import mipmap chains",
143 "intentionally true color"
146 new BlockField(
sizeof(bitmap::nested_block_test_entry),
"nested block test",
"testing block nesting",
150 new BlockField(
sizeof(bitmap::nested_block_test_entry::nested_block_child_block_entry),
"child block",
"",
Class representing an int16 tag field.
Definition: int.h:40
Namespace containing functions related to tag data.
Definition: bitmap.h:10
int width
Definition: bgfx.cpp:19
Class representing an ascii tag field.
Definition: ascii.h:12
Typedef for a bitfield32 field, used in tag data definitions.
Definition: tags.h:279
Class representing a data reference, or dataref for short.
Definition: dataref.h:14
Class representing a bitfield16 tag field.
Definition: bitfield.h:38
data_reference test_dataref
Definition: bitmap.h:74
Typedef for a bitfield8 field, used in tag data definitions.
Definition: tags.h:240
std::vector< BlamTagClassRevision > revisions
List of all tag class revisions. Does not get written to tags, but is included in plugin files.
Definition: tagclass.h:47
int integer_test
Definition: bitmap.h:22
Class representing an int8 tag field.
Definition: int.h:53
std::string class_name_short
The short, 4-character name of the tag class.
Definition: tagclass.h:45
int version
The tag class version. Should be incremented any time a tag class is modified whatsoever.
Definition: tagclass.h:46
Structure representing a Bitmap tag.
Definition: bitmap.h:20
Class representing a bitfield32 tag field.
Definition: bitfield.h:49
std::string class_name_long
The longer class name. Typically shown alongside short name for user-friendliness.
Definition: tagclass.h:44
TAG_BLOCK(bitmap_data, { short width;short height;byte depth;bitfield8 format_flags;TAG_ENUM(bitmap_type, { texture_2d, texture_3d, cubemap, unknown });})
BitmapTagClass()
Definition: bitmap.h:82
byte byte_test
Definition: bitmap.h:24
Class representing an int32 tag field.
Definition: int.h:27
Typedef for a bitfield16 field, used in tag data definitions.
Definition: tags.h:255
int height
Definition: bgfx.cpp:20
Structure representing a data reference.
Definition: tags.h:214
#define BLAM
Definition: discord_rpc.h:8
int tag_size
The size of the tag's data. Used on loading/writing tag files.
Definition: tagclass.h:51
BLAM bitmap * GetBitmapTag(std::string tag_path)
Definition: bitmap.cpp:5
Class representing a tag block field, also sometimes referred to as a struct or reflexive in the modd...
Definition: block.h:13
short short_test
Definition: bitmap.h:23
Class representing a tag class.
Definition: tagclass.h:41
std::vector< BlamPluginField * > fields
A series of tag fields that store the layout of the tag.
Definition: tagclass.h:49
Class representing a bitfield8 tag field.
Definition: bitfield.h:27