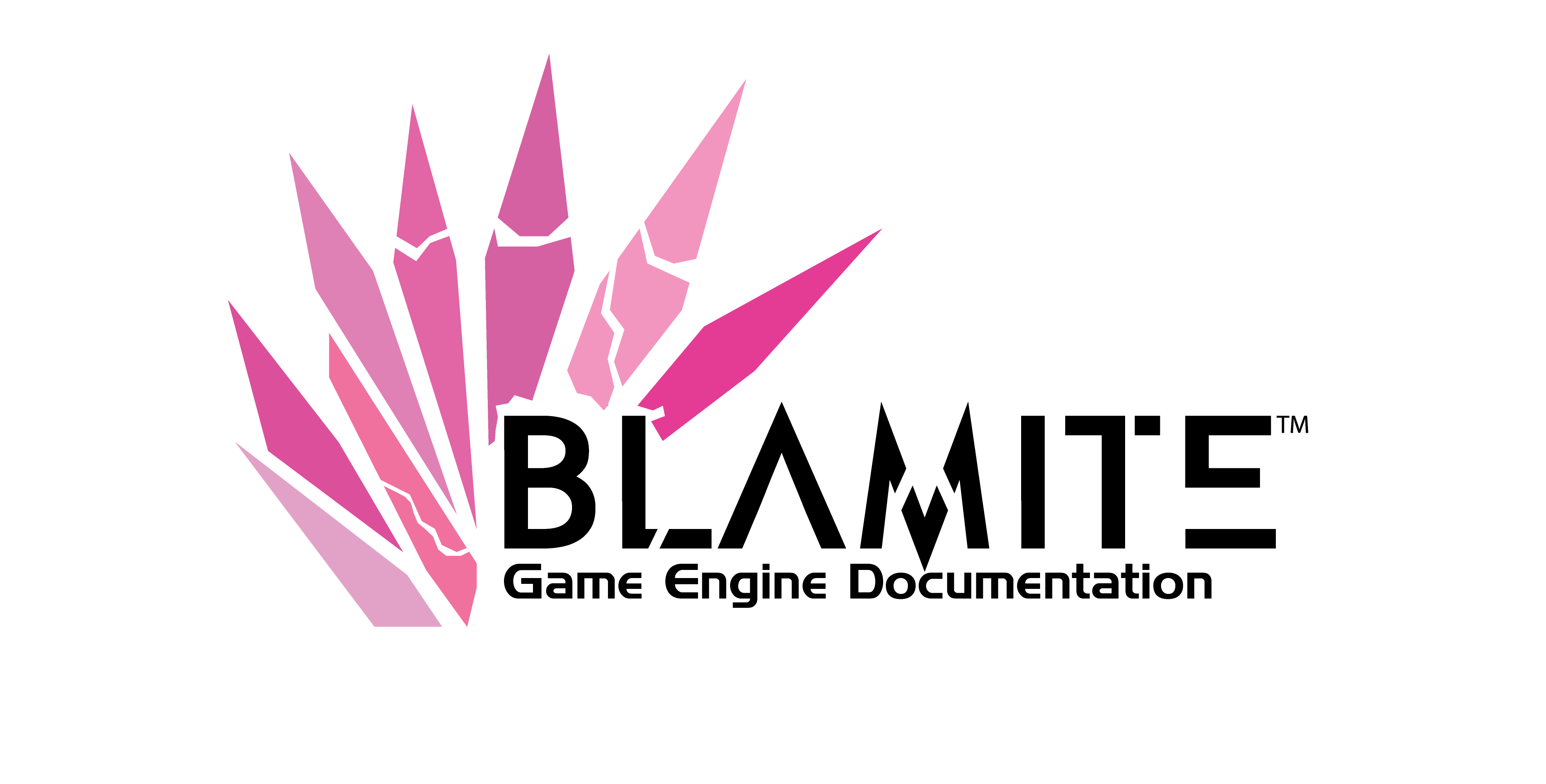 |
Blamite Game Engine - blam!
00346.12.11.21.0529.blamite
The core library for the Blamite Game Engine.
|
Go to the documentation of this file.
25 #include "../../imgui.h"
47 #if !defined(IMGUI_IMPL_OPENGL_ES2) \
48 && !defined(IMGUI_IMPL_OPENGL_ES3) \
49 && !defined(IMGUI_IMPL_OPENGL_LOADER_GL3W) \
50 && !defined(IMGUI_IMPL_OPENGL_LOADER_GLEW) \
51 && !defined(IMGUI_IMPL_OPENGL_LOADER_GLAD) \
52 && !defined(IMGUI_IMPL_OPENGL_LOADER_GLAD2) \
53 && !defined(IMGUI_IMPL_OPENGL_LOADER_GLBINDING2) \
54 && !defined(IMGUI_IMPL_OPENGL_LOADER_GLBINDING3) \
55 && !defined(IMGUI_IMPL_OPENGL_LOADER_CUSTOM)
58 #if defined(__APPLE__)
59 #include "TargetConditionals.h"
61 #if (defined(__APPLE__) && (TARGET_OS_IOS || TARGET_OS_TV)) || (defined(__ANDROID__))
62 #define IMGUI_IMPL_OPENGL_ES3 // iOS, Android -> GL ES 3, "#version 300 es"
63 #elif defined(__EMSCRIPTEN__)
64 #define IMGUI_IMPL_OPENGL_ES2 // Emscripten -> GL ES 2, "#version 100"
67 #elif defined(__has_include)
68 #if __has_include(<glew/glew.h>)
69 #define IMGUI_IMPL_OPENGL_LOADER_GLEW
70 #elif __has_include(<glad/glad.h>)
71 #define IMGUI_IMPL_OPENGL_LOADER_GLAD
72 #elif __has_include(<glad/gl.h>)
73 #define IMGUI_IMPL_OPENGL_LOADER_GLAD2
74 #elif __has_include(<GL/gl3w.h>)
75 #define IMGUI_IMPL_OPENGL_LOADER_GL3W
76 #elif __has_include(<glbinding/glbinding.h>)
77 #define IMGUI_IMPL_OPENGL_LOADER_GLBINDING3
78 #elif __has_include(<glbinding/Binding.h>)
79 #define IMGUI_IMPL_OPENGL_LOADER_GLBINDING2
81 #error "Cannot detect OpenGL loader!"
84 #define IMGUI_IMPL_OPENGL_LOADER_GL3W // Default to GL3W embedded in our repository
IMGUI_IMPL_API void ImGui_ImplOpenGL3_NewFrame()
Definition: imgui_impl_opengl3.cpp:238
IMGUI_IMPL_API void ImGui_ImplOpenGL3_DestroyFontsTexture()
Definition: imgui_impl_opengl3.cpp:475
IMGUI_IMPL_API void ImGui_ImplOpenGL3_Shutdown()
Definition: imgui_impl_opengl3.cpp:233
Add a fourth parameter to bake specific font ranges NULL
Definition: README.txt:57
IMGUI_IMPL_API bool ImGui_ImplOpenGL3_CreateFontsTexture()
Definition: imgui_impl_opengl3.cpp:446
IMGUI_IMPL_API bool ImGui_ImplOpenGL3_CreateDeviceObjects()
Definition: imgui_impl_opengl3.cpp:522
#define IMGUI_IMPL_API
Definition: imgui.h:60
IMGUI_IMPL_API bool ImGui_ImplOpenGL3_Init(const char *glsl_version=NULL)
Definition: imgui_impl_opengl3.cpp:153
IMGUI_IMPL_API void ImGui_ImplOpenGL3_RenderDrawData(ImDrawData *draw_data)
Definition: imgui_impl_opengl3.cpp:312
IMGUI_IMPL_API void ImGui_ImplOpenGL3_DestroyDeviceObjects()
Definition: imgui_impl_opengl3.cpp:702