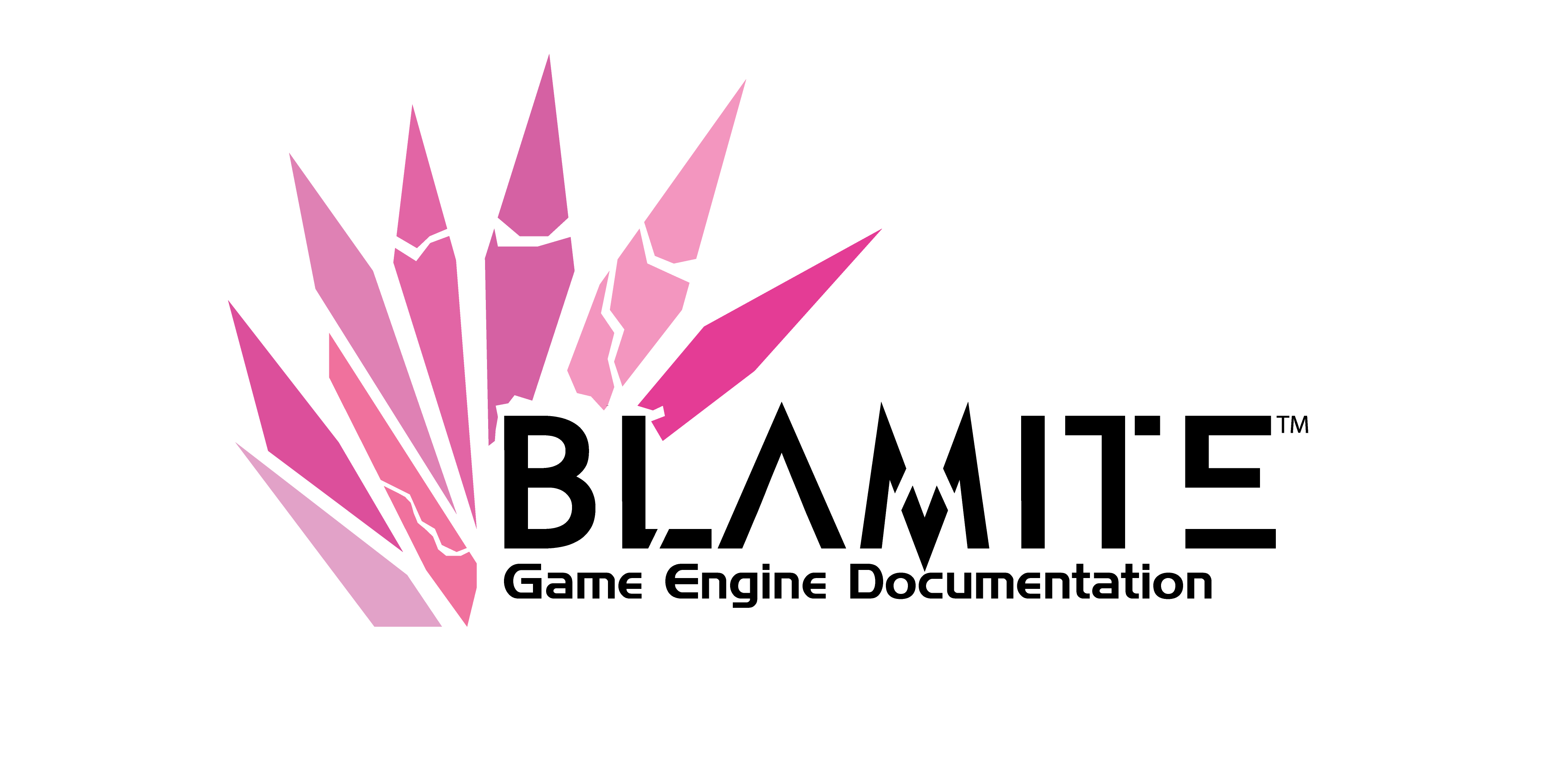 |
Blamite Game Engine - Guerilla (Library)
00386.06.16.23.0646.blamite
The tag editor for the Blamite Game Engine.
|
Go to the documentation of this file.
7 #ifdef GUERILLA_LIB_EXPORTS
8 #define GUERILLA_LIB_API __declspec(dllexport)
10 #define GUERILLA_LIB_API __declspec(dllimport)
39 std::string value =
"";
Class representing a tag field.
Definition: fields.h:202
@ Int16
Indicates that the field is a 16-bit integer.
std::string GetTagPath()
Generates the tag path, relative to its project.
Definition: BlamTag.cpp:704
BlamTagFieldType GetType()
Retrieves the type of this field.
Definition: BlamTagField.cpp:19
@ Real
Indicates that the field is a Float32.
virtual BlamTagField * GenerateTagField(BlamTag *tag)
Generates a new tag field from this plugin field.
Definition: BlamPluginField.cpp:40
@ Int32
Indicates that the field is a 32-bit integer.
std::string content_root
The root folder containing project content.
Definition: projects.h:94
void ReleaseFieldData()
Releases all items in fields.
Definition: BlamTag.cpp:402
Class representing an tagref plugin field.
Definition: tagref.h:20
int next_new_tag_number
The number to use for the next newly created tag.
Definition: BlamPlugin.cpp:23
int fieldset_version
The fieldset version of the tag.
Definition: tags.h:102
@ Enum8
Indicates that the field is an Enum8.
Class representing a plugin enum field.
Definition: enum.h:31
BlamProject * project
The project that this tag belongs to.
Definition: tags.h:259
std::string class_name_short
The short class name. Ex: scnr. Must be 4 characters or fewer.
Definition: tags.h:99
@ Vector4
Indicates that the field is a vector4.
std::vector< BlamBitfieldBitData > bits
The list of bits within this bitfield.
Definition: bitfield.h:67
~BlamPlugin()
Destroys the plugin data and releases all field data.
Definition: BlamPlugin.cpp:187
@ Block
Indicates that the field is a tag block.
BlamPluginField * ParseField(rapidxml::xml_node<> *field_node)
Attempts to parse an XML node as a plugin field.
Definition: BlamPlugin.cpp:77
std::vector< BlamTagBlockEntry * > entries
The list of entries within this block.
Definition: block.h:127
std::string description
The description of the revision.
Definition: tags.h:69
std::string author
The revision author.
Definition: tags.h:67
std::vector< BlamRevision > revisions
The list of revisions within the tag.
Definition: tags.h:254
@ Ascii
Indicates that the field is a string of text.
Class representing a vector plugin field.
Definition: vector.h:18
std::string field_id
The ID of this field.
Definition: fields.h:213
BlamVector4 value
The value of the field, represented as a 4D vector.
Definition: vector.h:40
bool IsBuiltIn()
Checks if the plugin is a built-in plugin.
Definition: BlamPlugin.cpp:468
@ Dataref
Indicates that the field is a data reference.
@ Enum16
Indicates that the field is an Enum16.
std::string GenerateXMLString(bool keep_unused, bool keep_invalid)
Generates a string with the field ID and value, ready to be written to an XML file.
Definition: BlamTagField_Block.cpp:133
Data structure representing a tag block entry.
Definition: block.h:17
@ Bitfield16
Indicates that the field is a Bitfield16.
BlamTagUpgradePolicy upgrade_policy
The tag's upgrade policy.
Definition: tags.h:271
BlamTagFieldType
Enumerator containing all possible tag field types.
Definition: fields.h:24
@ Int8
Indicates that the field is an 8-bit integer.
std::string id
The ID of the bit.
Definition: bitfield.h:30
BlamColor value
The value of the field, represented as a color.
Definition: color.h:38
std::vector< BlamRevision > revisions
The list of revisions contained within this plugin.
Definition: tags.h:104
Class representing a real (float32) tag field.
Definition: float32.h:32
std::vector< BlamPluginField * > fields
The list of fields contained within this plugin.
Definition: tags.h:105
void RecalculateFieldData()
Recalculates all offsets and lengths for the plugin.
Definition: BlamPlugin.cpp:401
Structure representing a tag or plugin revision.
Definition: tags.h:65
std::string referenced_tag_class
Definition: tagref.h:47
Class representing an integer plugin field.
Definition: int.h:16
Class representing a block plugin field.
Definition: block.h:66
@ Color
Indicates that the field is a color.
@ Comment
Indicates that the field is a comment. These do not store any tag data.
virtual std::string GenerateXMLString()
Generates a string with the field ID and value, ready to be written to an XML file.
Definition: BlamTagField.cpp:9
std::string file_path
The path to the plugin file.
Definition: tags.h:107
std::vector< BlamTagField * > fields
The list of fields within the tag.
Definition: tags.h:255
BlamResult LoadFromDisk()
Loads the tag as an XML document from disk.
Definition: BlamTag.cpp:425
int GetVersion()
Retrieves the current revision of the plugin.
Definition: BlamPlugin.cpp:307
BlamPlugin * plugin
Pointer to the plugin associated with this tag.
Definition: tags.h:252
Class representing an tagref tag field.
Definition: tagref.h:40
Class representing a color plugin field.
Definition: color.h:17
Clas representing a bitfield tag field.
Definition: bitfield.h:64
Class representing a block tag field.
Definition: block.h:124
Class representing a plugin field.
Definition: fields.h:60
Class representing a dataref tag field.
Definition: dataref.h:34
Class representing a Tag.
Definition: tags.h:232
bool SavePlugin(bool recalculate_field_data=true)
Saves the plugin file back to disk.
Definition: BlamPlugin.cpp:416
BlamTagField * GetField(std::string field_id)
Locates a tag field with a specific ID.
Definition: BlamTag.cpp:672
bool value
The bit's value.
Definition: bitfield.h:31
@ Int64
Indicates that the field is a 32-bit integer.
std::string current_option
The ID of the currently selected option.
Definition: enum.h:61
@ Tagref
Indicates that the field is a tag reference.
Class representing a dataref plugin field.
Definition: dataref.h:17
virtual std::string GenerateCppStructString(std::string line_prefix="")
Generates a string containing C++ code representing this plugin field.
Definition: BlamPluginField.cpp:74
bool HasPluginField()
Checks whether the field has plugin data available.
Definition: BlamTagField.cpp:34
virtual bool ParseXMLData(rapidxml::xml_node<> *field_node)
Populates data within the plugin field from an XML node.
Definition: BlamPluginField.cpp:84
int data_size
The size of the referenced data.
Definition: dataref.h:38
std::vector< BlamTagField * > fields
The list of fields within the entry.
Definition: block.h:21
@ Vector3
Indicates that the field is a vector3.
Class representing a tag enum field.
Definition: enum.h:58
int version
The revision version.
Definition: tags.h:68
float value
The value of the field.
Definition: float32.h:37
void SaveToDisk(std::string _file_path)
Saves any modifications to the XML tag back to disk.
Definition: BlamTag.cpp:519
Class representing an integer tag field.
Definition: int.h:32
int base_size
The base size of the tag.
Definition: tags.h:101
Class representing a vector tag field.
Definition: vector.h:35
BlamTag(std::string _file_path, BlamTagUpgradePolicy _upgrade_policy)
Prepares a new tag to be loaded.
Definition: BlamTag.cpp:412
Clas representing a bitfield plugin field.
Definition: bitfield.h:39
@ Bitfield8
Indicates that the field is a Bitfield8.
Class representing an ascii plugin field.
Definition: ascii.h:18
bool LocatePlugin()
Attempts to locate a compatiable plugin for a given tag.
Definition: BlamTag.cpp:640
BlamPlugin(std::string _file_path)
Prepares a new plugin for load.
Definition: BlamPlugin.cpp:177
void * data_address
The address of the referenced data.
Definition: dataref.h:37
BlamResult LoadFromDisk()
Loads the plugin XML document from disk.
Definition: BlamPlugin.cpp:192
Class representing a real (float32) plugin field.
Definition: float32.h:16
std::string class_name_long
The long class name. Ex: scenario. Can be any length.
Definition: tags.h:98
std::string file_path
The path to the tag file.
Definition: tags.h:257
std::string class_name
The class name as specified in the tag file. Used in the event a plugin could not be found.
Definition: tags.h:268
@ Unspecified
Indicates that the field's type is not specified. Fields with this type should be considered invalid.
#define TAG_FIELDSET_VERSION
BlamTag * CreateNewTag()
Creates a new tag using the plugin.
Definition: BlamPlugin.cpp:322
BlamPluginField * GetField(std::string id)
Attempts to locate a field with a given ID.
Definition: BlamPlugin.cpp:361
@ Bitfield32
Indicates that the field is a Bitfield32.
std::string name
The name of the entry.
Definition: block.h:20
@ Vector2
Indicates that the field is a vector2.
Data structure containing data for a bitfield bit.
Definition: bitfield.h:28
#define GUERILLA_LIB_API
Definition: ascii.h:10
int64_t value
The value of the field, represented as a 32-bit integer.
Definition: int.h:37
virtual std::string GenerateCppClassString(std::string line_prefix="")
Generates a string containing C++ code representing this plugin field.
Definition: BlamPluginField.cpp:79
bool ExportPluginToCpp()
Exports the plugin file to the appropriate C++ source and header files.
Definition: BlamPlugin.cpp:473
int class_version
The class/plugin version of the tag.
Definition: tags.h:267
virtual std::vector< char > GetValueAsBytes()
Retrieves the field value as a list of bytes.
Definition: BlamTagField.cpp:14
@ Enum32
Indicates that the field is an Enum32.
std::string referenced_tag_path
Definition: tagref.h:46
std::string value
The value of the field.
Definition: ascii.h:39
~BlamTag()
Releases any data used by the tag by calling ReleaseFieldData.
Definition: BlamTag.cpp:420
Class representing a color tag field.
Definition: color.h:33
Class representing an ascii tag field.
Definition: ascii.h:34